设计一个Car类,它的数据成员可以描述一辆汽车的品牌、型号、出厂日期和价格,成员函数要能提供合适的途径来访问数据成员,在main()函数中定义类的对象并调用相应成员函数。要求构造函数包括带参和不带参两种形式。
时间: 2024-11-05 11:34:23 浏览: 49
当设计一个`Car`类时,首先我们需要考虑的是数据成员(属性)以及它们的操作接口。根据题目要求,我们有以下几个数据成员:
- `string brand` - 品牌
- `string model` - 型号
- `string manufactureDate` - 出厂日期
- `double price` - 价格
接下来是成员函数(方法),包括构造函数(ctor)、访问器(getter)和可能的修改器(setter)。
```cpp
class Car {
public:
// 构造函数,带参数
Car(string brand = "", string model = "", string manufactureDate = "", double price = 0.0)
: brand(brand), model(model), manufactureDate(manufactureDate), price(price) {}
// 构造函数,无参数
Car() : brand(""), model(""), manufactureDate(""), price(0.0) {}
// 访问数据成员的方法(getter)
string getBrand() const { return brand; }
string getModel() const { return model; }
string getManufactureDate() const { return manufactureDate; }
double getPrice() const { return price; }
// 可选的修改数据成员的方法(setter)
void setBrand(string brand) { this->brand = brand; }
void setModel(string model) { this->model = model; }
void setManufactureDate(string manufactureDate) { this->manufactureDate = manufactureDate; }
void setPrice(double price) { this->price = price; }
private:
string brand;
string model;
string manufactureDate;
double price;
};
```
在`main()`函数中,我们可以创建一个`Car`对象并使用这些成员函数进行操作:
```cpp
int main() {
// 创建一个带参数的Car对象
Car myCar("Toyota", "Camry", "2020-01-01", 30000.0);
// 输出初始信息
cout << "Brand: " << myCar.getBrand() << endl;
cout << "Model: " << myCar.getModel() << endl;
cout << "Manufacture Date: " << myCar.getManufactureDate() << endl;
cout << "Price: " << myCar.getPrice() << endl;
// 修改数据成员后输出更新后的信息
myCar.setBrand("Honda");
myCar.setPrice(35000.0);
cout << "Updated Brand: " << myCar.getBrand() << endl;
cout << "Updated Price: " << myCar.getPrice() << endl;
return 0;
}
```
阅读全文
相关推荐
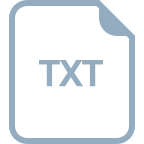
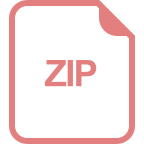
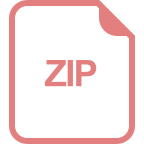






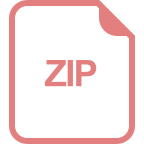
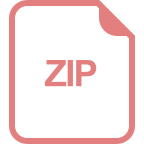
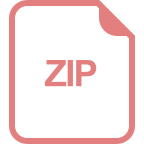
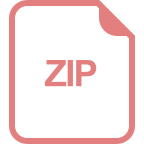
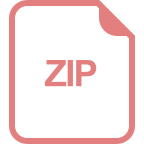
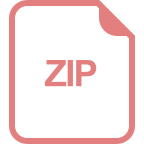
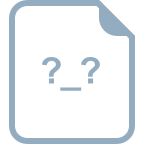
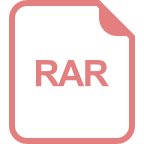
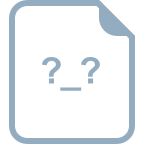