纯跟踪算法 python
时间: 2024-04-30 16:16:35 浏览: 9
纯跟踪算法(pure pursuit algorithm)是一种用于自主车辆导航的算法,它是基于车辆与路径之间的距离来计算车辆下一步要采取的行动。该算法基于假设,即路径是由一系列连续的线段组成的,并且车辆在每个时间步长内只能前进一段距离。在每个时间步长内,纯跟踪算法会计算车辆与路径之间的最短距离,并将车辆的目标点设置为路径上与车辆最近的点。然后,算法会计算车辆应该前进的距离和方向,并将这些信息作为控制器的输出。
以下是使用Python实现纯跟踪算法的示例代码:
``` python
import math
class PurePursuit:
def __init__(self, lookahead_distance):
self.lookahead_distance = lookahead_distance
def get_target_point(self, path, current_pos):
min_distance = float("inf")
target_point = None
for i in range(len(path)-1):
p1 = path[i]
p2 = path[i+1]
distance = self.distance_to_line(p1, p2, current_pos)
if distance < min_distance:
min_distance = distance
target_point = self.get_closest_point_on_line(p1, p2, current_pos)
if target_point is None:
target_point = path[-1]
return target_point
def calculate_steering(self, current_pos, current_heading, target_point):
dx = target_point[0] - current_pos
dy = target_point - current_pos
alpha = math.atan2(dy, dx) - current_heading
return alpha
def distance_to_line(self, p1, p2, p3):
return abs((p2-p1)*p3 - (p2-p1)*p3 + p2*p1 - p2*p1) / math.sqrt((p2-p1)**2 + (p2-p1)**2)
def get_closest_point_on_line(self, p1, p2, p3):
dx = p2 - p1
dy = p2 - p1
u = ((p3-p1)*dx + (p3-p1)*dy) / (dx*dx + dy*dy)
x = p1 + u*dx
y = p1 + u*dy
return x, y
def get_control(self, path, current_pos, current_heading):
target_point = self.get_target_point(path, current_pos)
alpha = self.calculate_steering(current_pos, current_heading, target_point)
distance = math.sqrt((target_point-current_pos[0])**2 + (target_point-current_pos)**2)
speed = distance / self.lookahead_distance
return speed, alpha
```
相关推荐
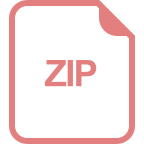
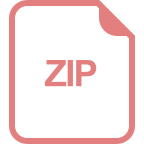














