在 main方法中实现数组的指定位置插入一条数组
时间: 2024-10-11 17:10:38 浏览: 9
在Java中,要在`main`方法中向数组的特定位置插入一个新的元素,可以按照以下步骤操作:
1. 定义一个数组并初始化部分元素。
2. 创建一个新元素(要插入的值)。
3. 计算插入后数组的新长度(当前长度加上1)。
4. 使用`System.arraycopy()`方法将原数组的部分元素向右移动一位。
5. 将新元素放置在计算出的位置。
下面是一个简单的示例代码片段,假设我们有一个整数数组`arr`,我们要在索引`index`处插入值`value`:
```java
public class Main {
public static void main(String[] args) {
// 初始化数组
int[] arr = {1, 2, 3, 4, 5};
int index = 2; // 插入位置
int value = 6; // 要插入的值
// 新长度
int newLength = arr.length + 1;
// 创建临时数组保存原数组的一部分
int[] temp = new int[newLength - 1];
// 移动元素到临时数组
System.arraycopy(arr, 0, temp, 0, index);
System.arraycopy(arr, index, temp, index, arr.length - index);
// 在目标位置插入新元素
temp[index] = value;
// 将临时数组复制回原数组
System.arraycopy(temp, 0, arr, 0, newLength);
// 打印更新后的数组
for (int i : arr) {
System.out.print(i + " ");
}
}
}
```
执行这段代码后,你会看到数组`arr`在索引`2`处插入了`6`,结果会是`1 2 6 3 4 5`。
相关推荐
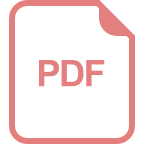
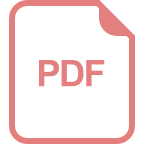
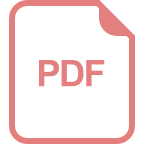















