``` # 这里加入车辆检测算法,例如YOLO或其他方法 detected_vehicles = detect_vehicles(frame) # 假设有个检测函数 ```帮助我实现车辆检测算法
时间: 2024-09-21 21:11:21 浏览: 56
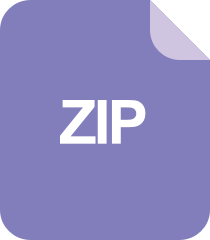
yolo算法-车辆数据集-788张图像带标签-自行车车vehicles_ped.zip
在Python中,要实现车辆检测算法,比如利用You Only Look Once (YOLO)方法,你需要先安装相关的库(如OpenCV、Darknet或TensorFlow-YOLOv3),然后编写以下步骤:
1. **导入必要的库**:
```python
import cv2
from yolov3.detect import YOLO # 假设有一个名为detect.py的YOLOV3模块
```
2. **加载预训练模型**:
```python
yolo = YOLO(weights_path='yolov3.weights', config_path='yolov3.cfg') # 需要YOLO的配置文件和权重文件路径
```
3. **读取视频帧或摄像头流**:
```python
cap = cv2.VideoCapture('video.mp4') # 或者0 for default camera
while True:
ret, frame = cap.read()
if not ret:
break
```
4. **执行车辆检测**:
```python
detected_vehicles = yolo.detect_objects(frame, threshold=0.5) # 检测并返回车辆的位置及其置信度
```
`detect_objects` 函数会应用YOLO的算法在给定帧上寻找车辆,并返回可能的车辆位置和其可信度。
5. **处理检测结果**:
```python
for vehicle in detected_vehicles:
x, y, w, h = vehicle['bbox']
label = vehicle['label'] # 可能是'car' or 'truck'
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2) # 在原图上绘制矩形框
cv2.putText(frame, label, (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
```
6. **显示带有标记的图像**:
```python
cv2.imshow('Vehicle Detection', frame)
key = cv2.waitKey(1)
if key == ord('q'):
break
```
完成上述代码后,你应该能看到实时的视频流中车辆被检测并标出。运行程序时记得检查模型文件路径是否正确。有关YOLO的具体细节,请查阅相应的教程或文档。如果你想要了解其他车辆检测方法(比如SSD、Faster R-CNN等),替换掉`yolov3.detect`的部分即可。
阅读全文
相关推荐
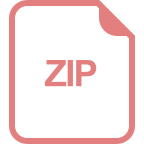



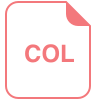
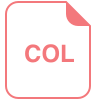
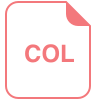

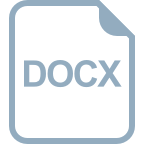
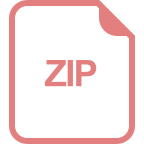
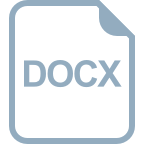
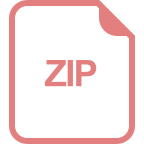
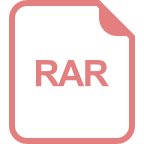
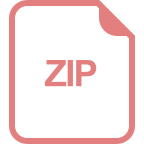
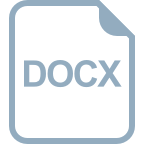