C#获取数组30到50位之间的最大值
时间: 2024-10-21 18:10:52 浏览: 26
在C#中,你可以通过以下步骤获取数组里30到50索引范围内的最大值:
1. 首先,假设你有一个整型数组`int[] array`。
2. 确保数组的长度大于等于30并且小于等于51,因为索引是从0开始的。
3. 使用LINQ查询来找到这个范围内的最大值。可以使用`Array.AsEnumerable()`将数组转换为`IEnumerable<int>`,然后利用`Where()`过滤出指定范围的元素,最后用`Max()`找出最大值。示例代码如下:
```csharp
int startIndex = 29; // 从0开始,30作为索引对应的位置
int endIndex = 49;
if (startIndex >= 0 && startIndex < array.Length && endIndex + 1 <= array.Length)
{
int maxInRange = array.Skip(startIndex).Take(endIndex - startIndex + 1)
.Max();
Console.WriteLine($"30到50位置的最大值是:{maxInRange}");
}
else
{
Console.WriteLine("数组的范围超出限制");
}
```
相关问题
C# 获取数组最大值
在C#中,获取数组的最大值可以使用几种方法。以下是其中的几种常见方法:
1. **Linq** (Language Integrated Query):如果你对.NET框架有更新版本的支持(如.NET Core),你可以使用`System.Linq`命名空间下的`Max()`函数来找到数组中的最大值。例如:
```csharp
int[] numbers = { 10, 5, 20, 15 };
int maxValue = numbers.Max();
```
2. **循环遍历**:如果没有使用Linq,你可以通过遍历数组并逐个比较元素来找出最大值。
```csharp
int[] numbers = { 10, 5, 20, 15 };
int maxValue = numbers[0];
foreach (int num in numbers)
{
if (num > maxValue)
maxValue = num;
}
```
3. **Array类的方法**:虽然`Max()`不是`Array`类直接提供的方法,但你可以自定义一个方法来实现:
```csharp
public static int Max(int[] array)
{
if (array == null || array.Length == 0)
throw new ArgumentException("Array must not be empty.");
return array.Max();
}
int[] numbers = { 10, 5, 20, 15 };
int maxValue = Max(numbers);
```
以上就是C#中获取数组最大值的一些常见方法。
C#获取数组最大值的索引
你可以使用以下代码来获取数组中最大值的索引:
```csharp
int[] array = { 1, 5, 3, 9, 2 };
int maxIndex = 0;
int maxValue = array[0];
for (int i = 1; i < array.Length; i++)
{
if (array[i] > maxValue)
{
maxValue = array[i];
maxIndex = i;
}
}
Console.WriteLine("最大值的索引: " + maxIndex);
```
在这个例子中,我们通过遍历数组来找到最大值,并记录其索引。初始时,假设第一个元素为最大值,然后我们逐个比较数组中的其他元素,如果遇到更大的值,就更新最大值和对应的索引。最后,我们输出最大值的索引。在这个例子中,最大值为9,索引为3。
阅读全文
相关推荐
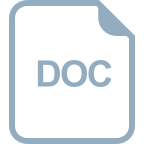
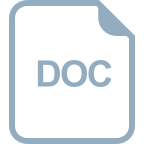
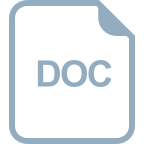
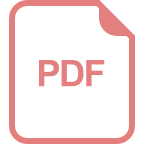
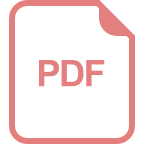


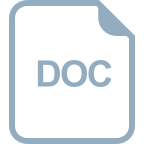








