js获取下一月的年月日
时间: 2024-11-12 15:34:08 浏览: 9
在JavaScript中,你可以使用`Date`对象和一些算术操作来获取当前日期的下一个月。以下是一个例子:
```javascript
// 获取当前日期
var currentDate = new Date();
// 提取当前月份和日期
var currentMonth = currentDate.getMonth() + 1; // JavaScript月份从0开始计数,加1转换成实际的月份
var currentDay = currentDate.getDate();
// 新的一年是从01月01日开始的,所以我们需要检查是否到了下一年
if (currentMonth === 13) {
// 如果当前已经是12月,增加一年
currentDate.setFullYear(currentDate.getFullYear() + 1);
currentMonth = 1;
}
// 设置新的日期为下个月的对应日期
currentDate.setDate(currentDay);
// 返回下个月的日期
console.log(currentDate.toDateString()); // 输出格式如 "Mon Dec 05 2022"
```
相关问题
js获取当前日期的下一月的年月日
在JavaScript中,获取当前日期的下一月的年月日,可以使用Date对象的`getMonth()`、`getDate()`和`getFullYear()`方法,然后通过增加一个月(注意不是简单加1),最后调整日期使其始终处于当月的最后一天。这是一个示例:
```javascript
function getNextMonthDate() {
const today = new Date();
let nextMonthDate = new Date();
// 如果今天已经是下个月的第一天,直接加一年
if (today.getDate() === 1) {
nextMonthDate.setFullYear(today.getFullYear() + 1);
} else {
nextMonthDate.setMonth(today.getMonth() + 1); // 加一个月
nextMonthDate.setDate(new Date(nextMonthDate.getFullYear(), nextMonthDate.getMonth()).getDate()); // 调整到当月最后一天
}
return nextMonthDate;
}
// 使用方法
const nextMonthAndDay = getNextMonthDate().toLocaleDateString('zh-CN', {day: '2-digit', month: 'long', year: 'numeric'}); // 返回格式如 "2023年二月 31日"
```
上述代码首先获取今天的日期,然后判断是否已经到了下个月第一天,如果是,则直接加一年;如果不是,就只加一个月,并确保日期是当月的最后一天。`toLocaleDateString()` 方法用于将日期格式化为本地化的字符串。
用js 获取 今日年月日时间 昨日年月日时间 当前年月 上个月年月
可以使用 JavaScript 的 Date() 对象来获取日期和时间。以下是获取今日年月日时间的代码:
```javascript
let today = new Date();
let year = today.getFullYear();
let month = today.getMonth() + 1;
let day = today.getDate();
let todayStr = year + '-' + month + '-' + day;
console.log(todayStr); // 输出格式为: 2022-10-14
```
获取昨日年月日时间可以通过减去一天的毫秒数来实现:
```javascript
let yesterday = new Date();
yesterday.setDate(yesterday.getDate() - 1);
let year = yesterday.getFullYear();
let month = yesterday.getMonth() + 1;
let day = yesterday.getDate();
let yesterdayStr = year + '-' + month + '-' + day;
console.log(yesterdayStr); // 输出格式为: 2022-10-13
```
获取当前年月可以使用类似获取今日年月日时间的代码:
```javascript
let today = new Date();
let year = today.getFullYear();
let month = today.getMonth() + 1;
let currentMonthStr = year + '-' + month;
console.log(currentMonthStr); // 输出格式为: 2022-10
```
获取上个月年月可以通过减去一个月的毫秒数来实现:
```javascript
let today = new Date();
today.setMonth(today.getMonth() - 1);
let year = today.getFullYear();
let month = today.getMonth() + 1;
let lastMonthStr = year + '-' + month;
console.log(lastMonthStr); // 输出格式为: 2022-09
```
阅读全文
相关推荐
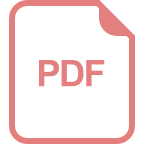
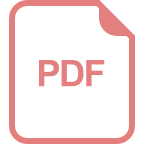
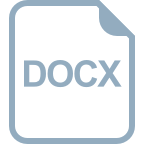





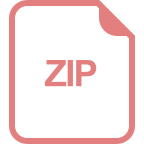







