如何编写一个hthreads程序
时间: 2024-09-09 19:16:33 浏览: 73
编写一个hthreads程序通常涉及以下步骤:
1. 定义线程函数:首先你需要定义线程将要执行的函数,这个函数将作为线程的入口点。在C语言中,这个函数通常需要符合一个特定的签名,比如在Linux平台上使用pthread时,其函数原型可能如下所示:
```c
void* thread_function(void* arg) {
// 线程要执行的代码
return NULL;
}
```
2. 创建线程:使用线程库提供的API创建新线程。例如,在pthread库中,你可以使用pthread_create()函数来创建线程:
```c
pthread_t thread_id;
pthread_create(&thread_id, NULL, thread_function, NULL);
```
3. 等待线程完成:如果你需要等待线程完成其任务并返回结果,可以使用pthread_join()函数:
```c
pthread_join(thread_id, NULL);
```
4. 销毁线程资源:当线程完成后,你可能需要清理相关资源。如果线程是在pthread库中创建的,当线程退出后,它的资源会被自动回收,不需要显式销毁。
这里是一个简单的例子,展示了如何在一个C程序中使用POSIX线程库(pthread)创建和运行一个线程:
```c
#include <stdio.h>
#include <pthread.h>
void* my_thread_function(void* arg) {
printf("Hello from the thread!\n");
return NULL;
}
int main() {
pthread_t thread_id;
// 创建线程
pthread_create(&thread_id, NULL, my_thread_function, NULL);
// 等待线程结束
pthread_join(thread_id, NULL);
printf("Thread finished execution.\n");
return 0;
}
```
阅读全文
相关推荐
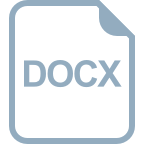
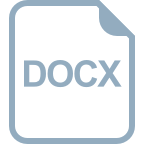
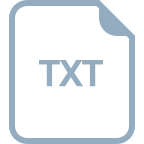
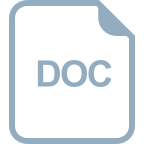



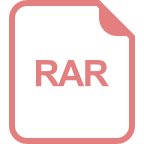
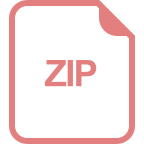
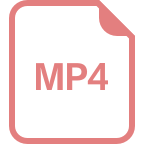
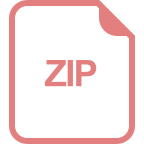
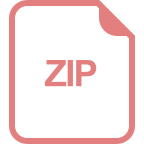
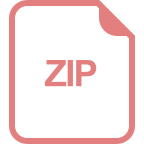