用java的GUI编写一个整数加法器,要求顶部按钮既可以清空也可以显示下方按钮按的数字及结果。
时间: 2024-10-29 08:24:43 浏览: 15
在Java中,我们可以使用Swing库来创建图形用户界面(GUI)来实现这个需求。以下是一个简单的例子:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class IntegerAdder extends JFrame {
private JTextField inputField1, inputField2;
private JButton button1, button2, displayResult;
private JLabel resultLabel;
private int sum = 0;
public IntegerAdder() {
setTitle("整数加法器");
setSize(400, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
setLayout(new GridLayout(5, 2));
inputField1 = new JTextField();
button1 = new JButton("输入");
button1.addActionListener(e -> handleInput(inputField1));
inputField2 = new JTextField();
button2 = new JButton("输入");
button2.addActionListener(e -> handleInput(inputField2));
displayResult = new JButton("显示结果");
displayResult.addActionListener(e -> displaySum());
resultLabel = new JLabel("结果: ");
resultLabel.setEditable(false);
panel.add(inputField1);
panel.add(button1);
panel.add(inputField2);
panel.add(button2);
panel.add(displayResult);
panel.add(resultLabel);
add(panel);
setVisible(true);
}
private void handleInput(JTextField textField) {
textField.setText("");
}
private void displaySum() {
try {
int num1 = Integer.parseInt(inputField1.getText());
int num2 = Integer.parseInt(inputField2.getText());
sum = num1 + num2;
resultLabel.setText("结果: " + sum);
} catch (NumberFormatException e) {
JOptionPane.showMessageDialog(this, "请输入有效的整数!");
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> new IntegerAdder());
}
}
```
在这个程序中,我们创建了一个`JFrame`作为窗口,并添加了两个`JTextField`用于输入数字,两个`JButton`分别用于输入操作和显示结果。当点击“显示结果”按钮时,会获取两个文本框的内容并计算总和,结果显示在`resultLabel`上。如果输入的不是整数,会在弹出框中提示。
阅读全文
相关推荐
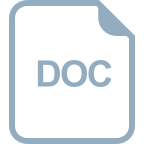
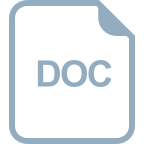
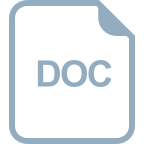
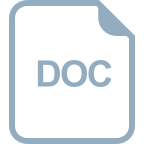
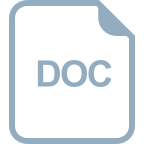
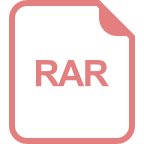
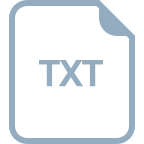
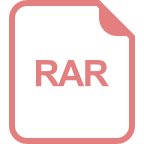
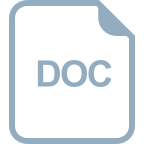
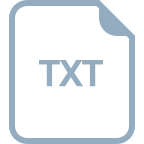
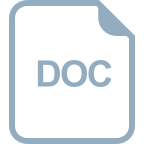
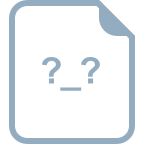
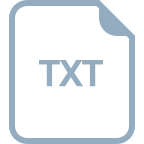
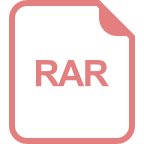
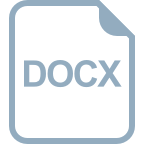
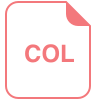

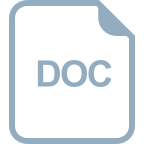
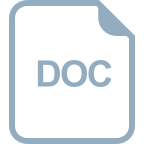