多线程 yolov5
时间: 2024-01-25 08:12:21 浏览: 129
多线程在yolov5中的应用可以通过使用线程池来实现。线程池可以帮助管理线程,避免为每个任务创建一个线程,提高系统的效率。
以下是一个使用线程池实现多线程yolov5目标检测的示例代码:
```python
import cv2
import torch
from torchvision import transforms
from yolov5.models.experimental import attempt_load
from yolov5.utils.general import non_max_suppression, scale_coords
from yolov5.utils.torch_utils import select_device
# 加载模型
weights = 'yolov5s.pt'
device = select_device('')
model = attempt_load(weights, map_location=device)
stride = int(model.stride.max())
# 定义目标类别
class_names = model.module.names if hasattr(model, 'module') else model.names
# 定义图像预处理函数
def preprocess_image(image):
img = transforms.ToTensor()(image).unsqueeze(0)
img = img.float() / 255.0
return img
# 定义目标检测函数
def detect_objects(image):
img = preprocess_image(image)
img = img.to(device)
# 进行目标检测
with torch.no_grad():
pred = model(img)[0]
pred = non_max_suppression(pred, conf_thres=0.5, iou_thres=0.5)
# 处理检测结果
detections = []
for det in pred:
if det is not None and len(det):
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], image.shape).round()
for *xyxy, conf, cls in reversed(det):
label = f'{class_names[int(cls)]} {conf:.2f}'
detections.append((label, xyxy))
return detections
# 定义多线程目标检测函数
def multi_thread_detection(image_list):
import concurrent.futures
# 创建线程池
num_threads = 4 # 设置线程数
executor = concurrent.futures.ThreadPoolExecutor(max_workers=num_threads)
# 提交任务到线程池
futures = []
for image in image_list:
future = executor.submit(detect_objects, image)
futures.append(future)
# 获取任务结果
results = []
for future in concurrent.futures.as_completed(futures):
result = future.result()
results.append(result)
return results
# 加载图像
image1 = cv2.imread('image1.jpg')
image2 = cv2.imread('image2.jpg')
image3 = cv2.imread('image3.jpg')
# 多线程目标检测
images = [image1, image2, image3]
detections = multi_thread_detection(images)
# 打印检测结果
for i, detection in enumerate(detections):
print(f'Detections for image {i+1}:')
for label, xyxy in detection:
print(label, xyxy)
```
这段代码首先加载了yolov5模型和相关的类别信息,然后定义了图像预处理函数和目标检测函数。接着,通过创建线程池并提交任务到线程池中,实现了多线程的目标检测。最后,打印了检测结果。
阅读全文
相关推荐
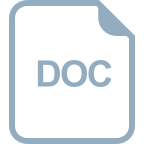
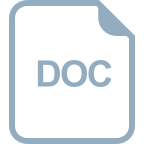
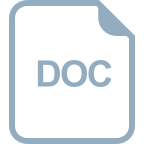
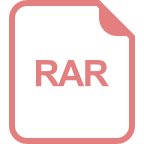
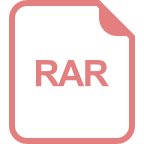
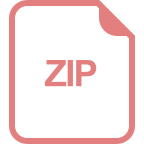
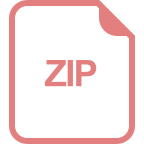











