编写程序,输入一个以$结束的字符串,要求过滤所有的非十六进制字符(不区分大小写),组成一个新的表示十六进制数字的字符串,然后将其转换为十进制数后输出。如果在第一个十六进制字符之前存在字符“-”,则代表该数是负数(输出格式:第一行输出十六进制的字符串,第二行输出转换后的十进制数)
时间: 2024-12-24 10:35:26 浏览: 8
要实现这个功能,你可以按照以下步骤编写C++代码:
1. 首先,读取用户输入的字符串直到遇到美元符号($)为止。
2. 检查字符串的第一个字符是否为减号("-"),如果是,则设置一个标志表示这是一个负数。
3. 接下来,遍历字符串,只保留十六进制字符(0-9, A-F或a-f)。可以使用`isxdigit`函数从C++ `<cctype>`库来进行判断。
4. 将过滤后的字符拼接成新的十六进制字符串。
5. 使用`std::stoul`或`std::stoll`函数(取决于是否包含负数)将十六进制字符串转换为无符号长整型(对于大数),如果需要处理负数,则用`std::stoi`或`std::stol`。
6. 输出结果。
以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <string>
#include <cctype>
int main() {
std::string input;
std::cout << "Enter a hexadecimal string (ends with $): ";
getline(std::cin, input, '$');
bool isNegative = input[0] == '-';
if (isNegative) {
input.erase(0, 1); // remove the first '-'
}
std::string hexStr;
for (char c : input) {
if (isxdigit(c)) { // check if character is a hexadecimal digit
hexStr += toupper(c); // convert to uppercase, if needed (for consistency)
}
}
long long decimalNum;
try {
decimalNum = isNegative ? -std::stoll(hexStr) : std::stoll(hexStr);
std::cout << "Hexadecimal string: " << hexStr << '\n';
std::cout << "Decimal number: " << decimalNum << '\n';
} catch (const std::invalid_argument& e) {
std::cerr << "Invalid hexadecimal number detected: " << hexStr << '\n';
return 1; // handle invalid input
}
return 0;
}
```
阅读全文
相关推荐
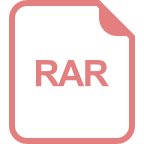
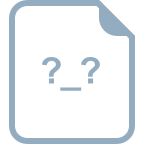
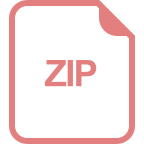


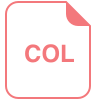
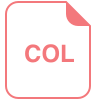
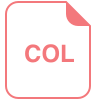
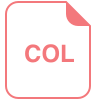
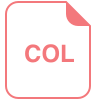
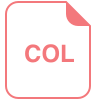
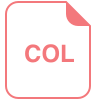
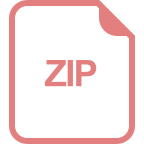
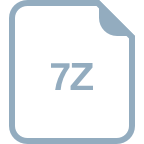
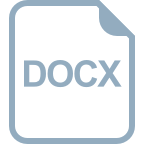
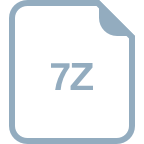