1. 简答题 给定一个存储整数的顺序表L1,试构造顺序表L2,并要求顺序表L2中只包含顺序表L1中所有值不相同的数据元素。给代码
时间: 2024-09-14 08:02:57 浏览: 77
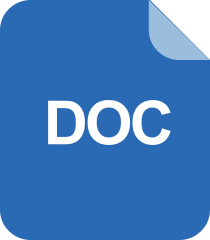
顺序表、链表试题库.doc
要构造一个顺序表L2,使得它包含顺序表L1中所有值不相同的数据元素,可以通过遍历顺序表L1,然后将每个新遇到的值添加到顺序表L2中。为了确保L2中元素的唯一性,我们需要在添加新元素前检查该元素是否已经在L2中存在。如果不存在,就添加它。下面是一个使用Python语言的示例代码,展示了如何实现这个过程:
```python
def create_unique_list(L1):
L2 = [] # 初始化L2为空列表
for item in L1:
if item not in L2: # 检查元素是否已在L2中
L2.append(item) # 如果不在,则添加到L2中
return L2 # 返回构造好的L2列表
# 示例使用
L1 = [1, 2, 3, 2, 4, 5, 3, 6]
L2 = create_unique_list(L1)
print(L2) # 输出应该是 [1, 2, 3, 4, 5, 6]
```
这段代码中,我们首先创建了一个空列表`L2`。然后,我们遍历列表`L1`中的每个元素,检查当前元素是否已经在`L2`中。如果不在,我们将其添加到`L2`中。最终,`L2`中将包含`L1`中的所有唯一元素。
阅读全文
相关推荐
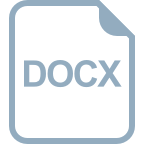
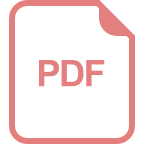

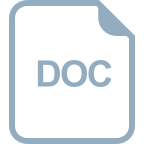
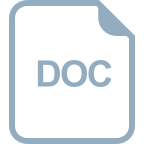

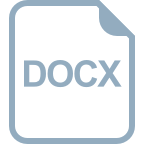
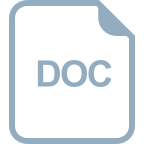
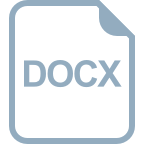
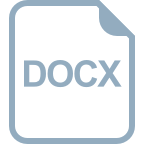
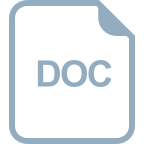
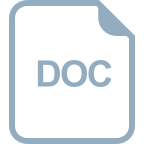
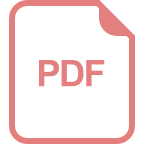
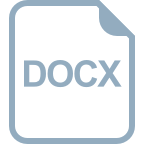
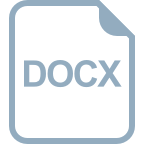
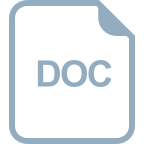
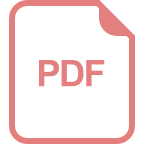
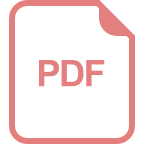