本关任务:实现 step1/SeqQueue.cpp 中的SQ_IsEmpty、SQ_IsFull、SQ_Length、SQ_In和SQ_Out五个操作函数,以实现判断队列是否为空、是否为满、求队列长度、队列元素入队和出队等功能。代码
时间: 2024-06-13 18:05:56 浏览: 258
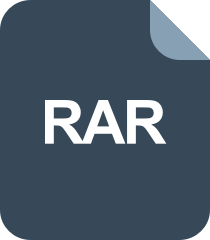
data_structure2.rar_seqqueue
以下是实现SQ_IsEmpty、SQ_IsFull、SQ_Length、SQ_In和SQ_Out五个操作函数的代码:
```cpp
#define MAXSIZE 100 // 定义队列的最大长度
typedef struct {
int data[MAXSIZE]; // 存储队列元素
int front; // 队头指针
int rear; // 队尾指针
} SeqQueue;
// 判断队列是否为空
bool SQ_IsEmpty(SeqQueue *Q) {
if (Q->front == Q->rear) {
return true;
} else {
return false;
}
}
// 判断队列是否为满
bool SQ_IsFull(SeqQueue *Q) {
if ((Q->rear + 1) % MAXSIZE == Q->front) {
return true;
} else {
return false;
}
}
// 求队列长度
int SQ_Length(SeqQueue *Q) {
return (Q->rear - Q->front + MAXSIZE) % MAXSIZE;
}
// 队列元素入队
bool SQ_In(SeqQueue *Q, int x) {
if (SQ_IsFull(Q)) {
return false;
} else {
Q->data[Q->rear] = x;
Q->rear = (Q->rear + 1) % MAXSIZE;
return true;
}
}
// 队列元素出队
bool SQ_Out(SeqQueue *Q, int *x) {
if (SQ_IsEmpty(Q)) {
return false;
} else {
*x = Q->data[Q->front];
Q->front = (Q->front + 1) % MAXSIZE;
return true;
}
}
```
阅读全文
相关推荐
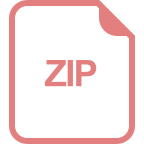















