用C语言写一个IIC协议
时间: 2024-11-15 12:21:07 浏览: 0
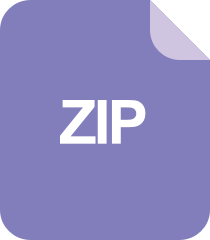
IIC协议用C语言实现

在C语言中实现I2C通信通常会依赖于硬件相关的库,如Linux的`i2c-dev.h`库或者针对特定微控制器的驱动。以下是一个简化的示例,展示了如何使用`i2c-dev.h`库通过系统调用在Linux平台上模拟基本的I2C发送和接收数据的过程。注意这只是一个基础框架,并未包括实际的传感器或设备的驱动。
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <linux/i2c-dev.h>
#define I2C_ADDR 0x40 // 设备地址
int main() {
int file;
char buffer[2] = {0}; // 数据发送数组
int ret;
// 打开I2C设备文件
if ((file = open("/dev/i2c-1", O_RDWR)) == -1) {
perror("Opening I2C device failed");
return 1;
}
// 设置I2C地址
if (ioctl(file, I2C_SLAVE, I2C_ADDR) < 0) {
perror("Setting slave address failed");
close(file);
return 1;
}
// 发送数据
buffer[0] = 0x01; // 假设我们要发送的数据
ret = write(file, buffer, sizeof(buffer));
if (ret < 0) {
perror("Writing data to I2C failed");
close(file);
return 1;
}
printf("Sent %d bytes\n", ret);
// 接收数据(这里仅作演示,实际应用需要先读取设备响应)
if (read(file, buffer, sizeof(buffer)) > 0) {
printf("Received: %02X\n", buffer[0]);
} else {
perror("Reading data from I2C failed");
}
close(file);
return 0;
}
```
这个程序首先打开I2C设备,设置目标地址,然后发送和接收数据。请注意,真正的I2C设备操作通常需要配合具体的中断处理和错误检查。
阅读全文
相关推荐
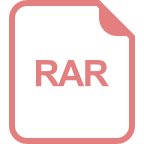
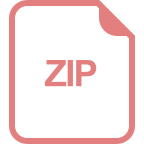







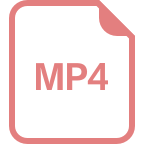
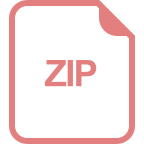
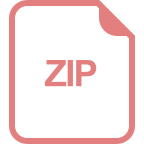
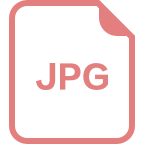