用C语言写硬件iic和模拟iic的区别举例
时间: 2024-10-19 16:14:30 浏览: 41
在C语言中,硬件IIC和模拟IIC的主要区别在于它们的底层实现和与物理硬件的直接交互。硬件IIC通常由专门的I2C接口芯片驱动,如MCP23S08或 PCA9546,直接与电子电路连接,而模拟IIC则是在软件层面上模拟I2C协议的行为。
硬件IIC代码示例(使用Linux的i2c-dev库):
```c
#include <stdio.h>
#include <linux/i2c.h>
int main() {
int fd = open("/dev/i2c-1", O_RDWR); // 打开I2C bus 1
if (fd < 0) {
perror("Opening I2C device");
return -1;
}
i2c_msg msg[] = {
{ I2C_M_WRITE, 0x76, &data, sizeof(data), NULL },
{ I2C_M_READ, 0x76, NULL, 1, &read_data }
};
int ret = i2c_transfer(fd, msg, 2);
if (ret != 2) {
perror("I2C transfer failed");
} else {
printf("Data sent: %s\n", hexdump(read_data));
}
close(fd);
return 0;
}
```
这里,我们通过文件描述符直接操作硬件I2C设备。
模拟IIC代码示例(例如在软件模拟环境中):
```c
#include <stdlib.h>
#include <string.h>
typedef struct {
uint8_t data[2];
} SimulatedI2CBus;
void simulate_write(SimulatedI2CBus* bus, uint8_t addr, const uint8_t* data, size_t len) {
// 这里仅做简单模拟,比如记录操作
memcpy(bus->data, data, len);
}
uint8_t* simulate_read(SimulatedI2CBus* bus, uint8_t addr, size_t* len) {
// 假设读取固定值
static uint8_t response[] = {0x42};
*len = sizeof(response);
return response;
}
// 使用模拟I2C函数
SimulatedI2CBus bus;
simulate_write(&bus, 0x76, data, sizeof(data));
```
总结:
1. **硬件IIC**更底层,直接操作硬件寄存器,对硬件配置有依赖,速度较快。
2. **模拟IIC**用于测试或软件环境,不需要真实硬件,便于调试,适合模拟复杂的通信场景。
阅读全文
相关推荐
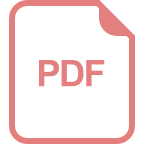
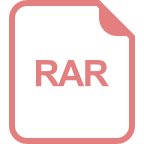
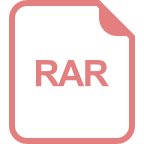




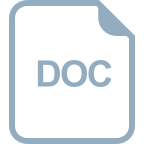
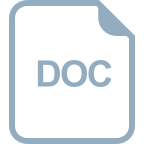




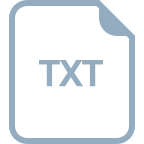
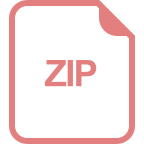
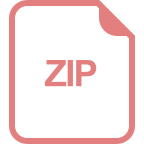
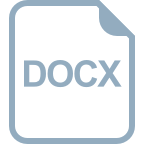
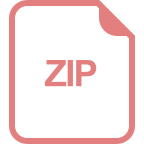