上述问题卷积神经网络python代码
时间: 2024-10-27 19:04:08 浏览: 24
如果你打算使用卷积神经网络 (CNN) 来处理火星风电场风机健康状态预测的问题,Python 中的一个流行库是 TensorFlow 或 PyTorch。这里我会给出一个基本的 Python 示例,使用 Keras 库,因为它构建在 TensorFlow 之上,而且易于理解和编写。假设我们已经有预处理过的数据 `X_train` 和 `y_train`(标签)以及 `X_test`(待预测的数据)。
```python
import numpy as np
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv1D, MaxPooling1D, Flatten, Dense
# 假设 X_train 是电气信号特征数组,y_train 是对应健康状态值,形状类似(样本数, 时间步长, 特征数)
# 我们先将数据转化为四维,以便 CNN 接受(时间步长, 特征数, 单位, 批次)
# 将数据标准化,方便网络学习
X_train = X_train.astype('float32') / 255.0
X_test = X_test.astype('float32') / 255.0
# 假设时间步长为 timesteps,特征数为 features,类别数为 num_classes(健康状态值的数量)
timesteps, features = X_train.shape[1], X_train.shape[2]
num_classes = len(np.unique(y_train))
# 构建 CNN 模型
model = Sequential([
Conv1D(filters=32, kernel_size=3, activation='relu', input_shape=(timesteps, features)),
MaxPooling1D(pool_size=2),
Conv1D(filters=64, kernel_size=3, activation='relu'),
MaxPooling1D(pool_size=2),
Flatten(), # 展平卷积层后的输出以便全连接层处理
Dense(128, activation='relu'),
Dense(num_classes, activation='softmax') # 输出层使用 softmax 函数,因为这是一个分类问题
])
# 编译模型
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# 训练模型
history = model.fit(X_train, y_train, epochs=10, validation_data=(X_test, y_test))
# 预测未知风机健康状态值
predictions = model.predict(X_test)
```
注意这只是一个基础示例,实际应用中可能还需要调整网络架构(如添加更多卷积层、池化层、Dropout 层等)、调整超参数,以及进行数据增强来提高模型性能。
阅读全文
相关推荐
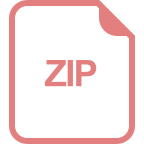
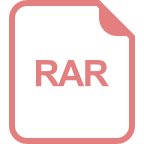
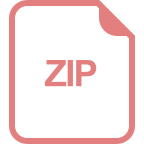



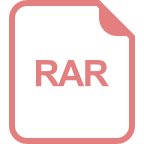
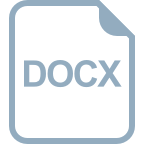
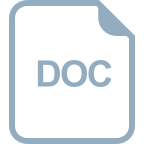
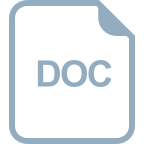








