c语言挑出数组中的最大值
时间: 2023-11-18 16:04:01 浏览: 142
可以使用循环遍历数组,依次比较每个元素的大小,找出最大值。具体实现可以参考以下代码:
```c
#include <stdio.h>
int main() {
int arr[] = {1, 5, 3, 9, 2};
int len = sizeof(arr) / sizeof(arr[0]); // 数组长度
int max = arr[0]; // 假设第一个元素为最大值
for (int i = 1; i < len; i++) {
if (arr[i] > max) {
max = arr[i]; // 更新最大值
}
}
printf("数组中的最大值为:%d\n", max);
return 0;
}
```
相关问题
用C语言挑出ADC采集到的最大数的程序,去掉数组,且18秒后自动结束循环,然后接收到来自上位机的一段特定的报文,可以再次循环
在C语言中,创建这样一个程序需要几个步骤。首先,你需要初始化一个变量作为当前最大值,然后在一个无限循环中不断检查新的ADC读数,直到达到设定的时间限制。如果接收到特定的报文,你可以中断循环并开始一个新的周期。
以下是一个基本的伪代码框架:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h> // 引入时间库
// 假设ADC读取函数为read_ADC()
unsigned short int current_max = 0;
void check_for_stop_signal(char stop_code) {
// 检查上位机发送的stop_code
// 这里只是一个简化示例,实际可能需要通过串口通信或其他接口解析数据
if (stop_code == 'S') { // 假设上位机发来的停止信号为'S'
end_loop();
}
}
void update_max_and_check_time(unsigned short int new_value) {
if (new_value > current_max) {
current_max = new_value;
}
// 检查18秒是否已过
struct timespec now;
clock_gettime(CLOCK_MONOTONIC, &now);
long elapsed_seconds = now.tv_sec - last_check_time;
if (elapsed_seconds >= 18) {
last_check_time = now.tv_sec; // 更新上次检查时间
check_for_stop_signal(stop_code_from_upper_side()); // 检查是否有停止信号
}
}
void end_loop() {
while (!stop_condition()) {
break; // 结束循环
}
start_new_loop(); // 如果有停止信号,这里可以开始新的循环周期
}
int main() {
struct timespec last_check_time = {0, 0}; // 初始化检查时间
while (true) {
unsigned short int new_value = read_ADC(); // 假设此函数返回新读取的ADC值
update_max_and_check_time(new_value);
// 18秒未到或有停止信号,继续等待
}
return 0;
}
```
请注意,这只是一个简化版的框架,实际实现可能需要包含更多的错误处理和细节。例如,你可能需要管理一个标志来判断是否应该继续循环,以及具体的ADC读取、停止信号检测以及与上位机的数据交互机制。
三个数,挑选五次,每次任意挑出一个数加1,求最大乘积,c语言暴力求解
为了实现C语言暴力求解三个数(假设为`a`, `b`, 和 `c`)在最多五次操作(每次加1)后的最大乘积,可以使用动态规划的方法。由于限制了操作次数,我们可以枚举每一次操作应用到哪个数,然后更新可能的最大乘积。
以下是C语言代码的一个示例[^1]:
```c
#include <stdio.h>
int main() {
int a, b, c, maxProduct = 0; // 初始化最大乘积为0
int operations = 5; // 最多操作5次
printf("Enter three numbers (a, b, c): ");
scanf("%d %d %d", &a, &b, &c); // 输入三个数
// 动态规划数组,dp[i][j]表示在i次操作后,a*b*c的可能最大乘积
int dp[6][3] = {{a * b * c}, {a * (b + 1) * c}, {a * b * (c + 1)},
{a * (b + 1) * (c + 1)}, {a * b * (c + 2)}, {a * (b + 1) * (c + 2)}};
// 比较每次操作的结果,找到最大乘积
for (int i = 0; i < operations; ++i) {
if (dp[i][0] > dp[i][1]) {
dp[i+1][0] = dp[i][0];
} else {
dp[i+1][0] = dp[i][1];
}
if (dp[i][0] > dp[i][2]) {
dp[i+1][1] = dp[i][0];
} else {
dp[i+1][1] = dp[i][2];
}
if (dp[i][1] > dp[i][2]) {
dp[i+1][2] = dp[i][1];
} else {
dp[i+1][2] = dp[i][2];
}
}
// 找到最后一次操作后最大乘积
maxProduct = dp[operations - 1][0]; // 取最后一次操作的可能最大值
printf("The maximum product after %d operations is: %d\n", operations, maxProduct);
return 0;
}
```
阅读全文
相关推荐
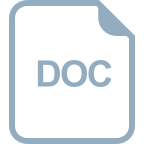
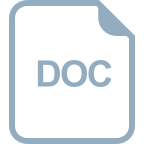
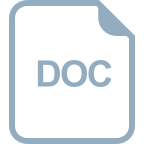
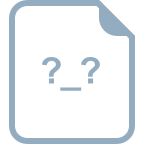
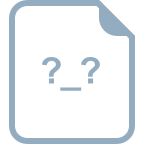
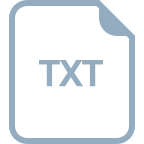
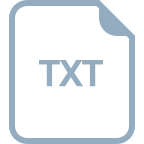
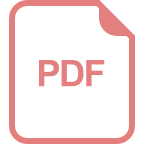
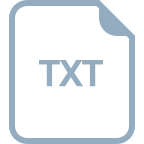
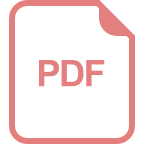
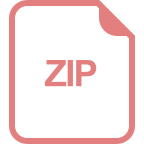
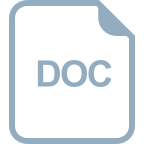
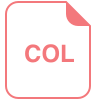
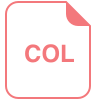

