Android 流式语音识别
时间: 2023-11-21 22:57:56 浏览: 38
基于Java SDK实现的Android实时音频流识别的Demo应用可以实现Android流式语音识别。具体步骤如下:
1.在Android Studio中创建一个新项目。
2.在app/build.gradle文件中添加以下依赖项:
```gradle
implementation 'com.baidu.aip:speech-sdk-android:3.6.6.5'
```
3.在AndroidManifest.xml文件中添加以下权限:
```xml
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.INTERNET" />
```
4.在MainActivity.java文件中添加以下代码:
```java
import com.baidu.speech.EventListener;
import com.baidu.speech.asr.SpeechConstant;
import com.baidu.speech.asr.SpeechRecognizer;
import com.baidu.speech.asr.SpeechResult;
import com.baidu.speech.asr.SpeechSynthesizeBag;
import com.baidu.speech.asr.SpeechUtility;
import com.baidu.speech.event.EventManager;
import com.baidu.speech.event.EventManagerFactory;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.Map;
public class MainActivity extends AppCompatActivity implements EventListener {
private EventManager asr;
private SpeechRecognizer speechRecognizer;
private boolean isRecording = false;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 初始化语音识别
SpeechUtility.createUtility(this, SpeechConstant.APPID + "=你的App ID");
// 创建语音识别EventManager
asr = EventManagerFactory.create(this, "asr");
asr.registerListener(this);
// 创建SpeechRecognizer
speechRecognizer = SpeechRecognizer.createSpeechRecognizer(this);
speechRecognizer.setRecognitionListener(new RecognitionListener() {
@Override
public void onReadyForSpeech(Bundle params) {
// 准备就绪,可以开始说话
}
@Override
public void onBeginningOfSpeech() {
// 开始说话
}
@Override
public void onRmsChanged(float rmsdB) {
// 音量变化
}
@Override
public void onBufferReceived(byte[] buffer) {
// 录音数据回调
}
@Override
public void onEndOfSpeech() {
// 结束说话
}
@Override
public void onError(int error) {
// 识别出错
}
@Override
public void onResults(Bundle results) {
// 识别结果回调
ArrayList<String> nbest = results.getStringArrayList(SpeechRecognizer.RESULTS_RECOGNITION);
if (nbest != null && nbest.size() > 0) {
String text = nbest.get(0);
// 处理识别结果
}
}
@Override
public void onPartialResults(Bundle partialResults) {
// 临时识别结果回调
}
@Override
public void onEvent(int eventType, Bundle params) {
// 处理事件回调
}
});
}
// 开始录音
private void startRecording() {
if (!isRecording) {
isRecording = true;
Map<String, Object> params = new LinkedHashMap<>();
params.put(SpeechConstant.ACCEPT_AUDIO_VOLUME, false);
params.put(SpeechConstant.VAD_ENDPOINT_TIMEOUT, 0);
params.put(SpeechConstant.VAD, SpeechConstant.VAD_DNN);
params.put(SpeechConstant.PID, 1537);
params.put(SpeechConstant.LANGUAGE, "zh");
params.put(SpeechConstant.NLU, "enable");
params.put(SpeechConstant.VAD_ENDPOINT_TIMEOUT, 800);
params.put(SpeechConstant.VAD, SpeechConstant.VAD_DNN);
params.put(SpeechConstant.DISABLE_PUNCTUATION, false);
params.put(SpeechConstant.ACCEPT_AUDIO_DATA, true);
params.put(SpeechConstant.OUT_FILE, "/sdcard/baiduASR/outfile.pcm");
String json = new JSONObject(params).toString();
asr.send(SpeechConstant.ASR_START, json, null, 0, 0);
speechRecognizer.startListening(new Intent());
}
}
// 停止录音
private void stopRecording() {
if (isRecording) {
isRecording = false;
speechRecognizer.stopListening();
asr.send(SpeechConstant.ASR_STOP, null, null, 0, 0);
}
}
@Override
public void onEvent(String name, String params, byte[] data, int offset, int length) {
if (name.equals(SpeechConstant.CALLBACK_EVENT_ASR_READY)) {
// 识别引擎准备就绪
} else if (name.equals(SpeechConstant.CALLBACK_EVENT_ASR_PARTIAL)) {
// 临时识别结果
try {
JSONObject jsonObject = new JSONObject(params);
String result = jsonObject.getString("results_recognition");
// 处理临时识别结果
} catch (JSONException e) {
e.printStackTrace();
}
} else if (name.equals(SpeechConstant.CALLBACK_EVENT_ASR_FINISH)) {
// 识别结束
}
}
}
```
5.在MainActivity.xml文件中添加一个按钮,用于开始和停止录音。
```xml
<Button
android:id="@+id/btn_record"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="开始录音"
android:onClick="onRecordButtonClick" />
```
6.在MainActivity.java文件中添加以下代码,用于处理按钮点击事件:
```java
public void onRecordButtonClick(View view) {
Button button = (Button) view;
if (isRecording) {
stopRecording();
button.setText("开始录音");
} else {
startRecording();
button.setText("停止录音");
}
}
```
7.运行应用程序并单击“开始录音”按钮,开始录制音频。当你停止录音时,应用程序将自动将音频发送到百度云进行语音识别,并返回识别结果。
相关推荐
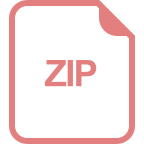














