VBA 正则表达式 用于匹配重复英文单词
时间: 2024-10-14 17:05:46 浏览: 56
VBA中的正则表达式是一种强大的文本处理工具,可以用来匹配、查找和替换复杂的模式。如果你想在VBA中使用正则表达式来匹配重复的英文单词,你可以创建一个模式来识别连续出现的相同单词。例如,模式`"\b(\w+)\b(?=\s+\1)"`可以做到这一点:
- `\b` 表示单词边界,确保我们只匹配整个单词。
- `(\w+)` 是一个捕获组,匹配一个或多个字母数字字符(等效于[a-zA-Z0-9_]),并存储到组1中。
- `(?=\s+\1)` 是一个向前前瞻,检查是否有空格后跟相同的组1内容,如果存在就认为找到了重复。
在VBA中,你可以这样使用:
```vba
Dim regex As Object
Set regex = CreateObject("vbscript.regexp")
regex.Pattern = "\b(\w+)\b(?=\s+\1)"
Dim text As String
text = "This is a test test, with repeated words."
Dim matches() As MatchCollection
Set matches = regex.Execute(text)
For Each match In matches
Debug.Print "Found duplicate: " & match.SubMatches(0) ' 输出找到的重复单词
Next match
```
相关问题
VBA 正则表达式 用于匹配连续标点符号后的英文单词
在VBA中,如果你想要匹配连续标点符号后的英文单词,可以使用正则表达式来识别并移除它们。下面是一个例子,展示了如何构建这样一个正则表达式和相应的VBA函数:
首先,创建一个`RegExp`对象,指定模式,该模式会查找由连续标点符号分隔的英文单词:
```vba
Dim regex As Object
Set regex = CreateObject("VBScript.Regexp")
regex.Pattern = "[^a-zA-Z]*\b\w+\b[^a-zA-Z]*" ' 这里[^a-zA-Z]表示非字母字符
```
在这个模式中,`\b\w+\b` 是匹配一个英文单词的部分,而`[^a-zA-Z]*` 匹配零个或多个非字母字符,即允许连续的标点符号存在。
接下来,编写一个函数,将满足条件的单词提取出来:
```vba
Function GetWordsWithPunctuation(strInput As String) As String
Dim match As MatchCollection
If Not regex.Test(strInput) Then
GetWordsWithPunctuation = strInput ' 如果没有匹配,则直接返回原字符串
Else
Set match = regex.Execute(strInput)
Dim word As String
Dim result As String
For Each word In match
result = result & Replace(word.SubString(word.Index, word.Length), word, "") ' 移除匹配的部分
Next
GetWordsWithPunctuation = result
End If
End Function
```
这个函数会在输入的字符串中查找所有匹配项,然后将其替换为空字符串,只保留其他部分。
最后,你可以像这样调用此函数:
```vba
Sub ProcessText()
Dim str As String
str = "Hello, world! This is a sample text with some punctuation., ."
Debug.Print GetWordsWithPunctuation(str)
End Sub
```
运行`ProcessText`后,你会得到如"Hello"这样的单词,标点符号已被移除。
vba正则表达式做模糊匹配
在 VBA 中,可以使用正则表达式实现模糊匹配。下面是一个示例代码,演示如何使用正则表达式进行模糊匹配:
```vba
Sub RegexFuzzyMatchExample()
Dim regex As Object
Dim match As Object
Dim inputString As String
' 创建正则表达式对象
Set regex = CreateObject("VBScript.RegExp")
' 设置正则表达式模式
regex.Pattern = "Hel+o"
' 设置要匹配的字符串
inputString = "Hello, Heeello, Helllo"
' 执行匹配操作
For Each match In regex.Execute(inputString)
' 输出匹配结果
MsgBox "Matched: " & match.Value
Next match
' 释放对象
Set match = Nothing
Set regex = Nothing
End Sub
```
上述示例中,我们创建了一个正则表达式对象 `regex`,设置了要匹配的模式为 `Hel+o`,表示匹配以 "H" 开头,后跟一个或多个 "e",最后以 "o" 结尾的单词。然后我们使用 `regex.Execute` 方法对输入字符串进行匹配,并通过循环遍历每个匹配项,输出匹配结果。
这里使用的模式是一个简单的示例,你可以根据自己的需求修改正则表达式模式来实现更复杂的模糊匹配逻辑。
希望这个示例对你有所帮助!如果你有任何进一步的问题,请随时提问。
阅读全文
相关推荐
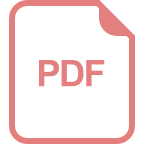
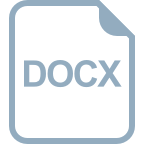
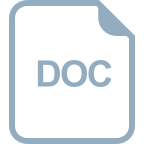
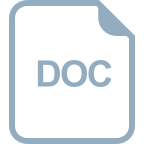


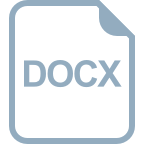
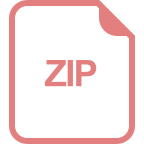
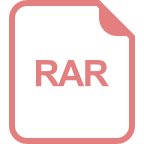
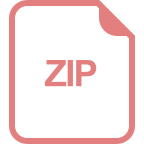
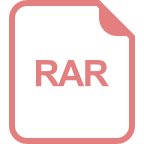
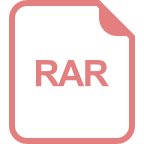
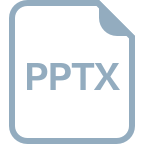
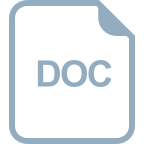
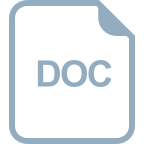

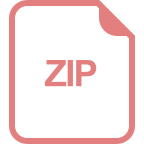