vue3 父子组件调用
时间: 2023-12-29 11:05:20 浏览: 28
以下是Vue3中父子组件之间的调用方法:
1. 父组件给子组件传参:
在父组件中使用props属性将数据传递给子组件,在子组件中通过props接收数据。
```vue
// 父组件
<template>
<div>
<child-component :message="message"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
data() {
return {
message: 'Hello from parent component'
};
}
};
</script>
// 子组件
<template>
<div>
<p>{{ message }}</p>
</div>
</template>
<script>
export default {
props: ['message']
};
</script>
```
2. 父组件调用子组件内的方法:
在子组件中使用ref属性给子组件命名,然后在父组件中通过$refs来调用子组件的方法。
```vue
// 父组件
<template>
<div>
<child-component ref="child"></child-component>
<button @click="callChildMethod">Call Child Method</button>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
methods: {
callChildMethod() {
this.$refs.child.childMethod();
}
}
};
</script>
// 子组件
<template>
<div>
<p>Child Component</p>
</div>
</template>
<script>
export default {
methods: {
childMethod() {
console.log('Child method called');
}
}
};
</script>
```
3. 子组件调用父组件的方法:
在父组件中使用v-on指令给子组件绑定一个自定义事件,在子组件中通过$emit方法触发该事件,并传递数据给父组件。
```vue
// 父组件
<template>
<div>
<child-component @custom-event="handleCustomEvent"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
methods: {
handleCustomEvent(data) {
console.log('Custom event received:', data);
}
}
};
</script>
// 子组件
<template>
<div>
<button @click="emitCustomEvent">Emit Custom Event</button>
</div>
</template>
<script>
export default {
methods: {
emitCustomEvent() {
this.$emit('custom-event', 'Custom data');
}
}
};
</script>
```
相关推荐
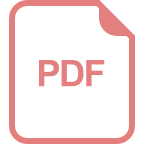














