java实现lpf转svg
时间: 2023-09-13 20:03:55 浏览: 46
要将LPF(Layout Parasitic File)转换为SVG(Scalable Vector Graphics),你可以使用Java编程语言,并借助一些开源库来实现。下面是一个示例代码,使用了Apache Batik库来进行LPF到SVG的转换:
首先,确保你已经引入了Apache Batik库的相关依赖。然后,你可以使用以下代码实现LPF到SVG的转换:
```java
import org.apache.batik.dom.svg.SVGDOMImplementation;
import org.apache.batik.svggen.SVGGraphics2D;
import org.w3c.dom.DOMImplementation;
import org.w3c.dom.Document;
import java.awt.*;
import java.awt.geom.AffineTransform;
import java.io.*;
public class LPFToSVGConverter {
public static void convertLPFToSVG(String lpfFilePath, String svgFilePath) throws IOException {
// Create a DOM document for SVG
DOMImplementation domImpl = SVGDOMImplementation.getDOMImplementation();
String svgNS = SVGDOMImplementation.SVG_NAMESPACE_URI;
Document document = domImpl.createDocument(svgNS, "svg", null);
// Create an SVGGraphics2D object to draw the LPF content
SVGGraphics2D svgGenerator = new SVGGraphics2D(document);
// Read the LPF file and draw its content to SVGGraphics2D
readLPFFile(lpfFilePath, svgGenerator);
// Finally, write the SVG document to the output file
writeSVGToFile(svgGenerator, svgFilePath);
}
private static void readLPFFile(String lpfFilePath, Graphics2D graphics) throws IOException {
BufferedReader reader = new BufferedReader(new FileReader(lpfFilePath));
String line;
while ((line = reader.readLine()) != null) {
// Process each line and draw the content to the SVGGraphics2D
// You may need to parse the LPF content and convert it to appropriate SVG elements
// For simplicity, let's just draw each line as text in the SVG file
graphics.drawString(line, 10, 10);
}
reader.close();
}
private static void writeSVGToFile(Graphics2D graphics, String svgFilePath) throws IOException {
OutputStream outputStream = new FileOutputStream(svgFilePath);
Writer out = new OutputStreamWriter(outputStream, "UTF-8");
graphics.stream(out, true); // Write the SVG document to the output stream
out.flush();
out.close();
outputStream.close();
}
public static void main(String[] args) {
try {
convertLPFToSVG("path/to/lpf/file.lpf", "path/to/svg/file.svg");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请注意,上述代码只是一个示例,你需要根据LPF文件的内容结构进行适当的解析和转换,将LPF元素映射到SVG元素。你可能还需要使用更复杂的绘图和转换逻辑来处理LPF文件的内容。
相关推荐
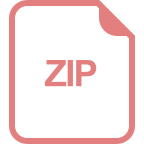














