文档中是我的Androidstudio中注册页面的代码,我现在要是注册页面功能,但现在有一个问题是我无法点击按钮或者说点击按钮时无反应,请你务必帮我解决这个问题并将正确的代码反馈给我,对其进行检查纠错,要求首先将问题分条详细列出,然后提供完整的更正后代码
时间: 2024-12-08 08:24:58 浏览: 13
### 问题分析
根据您提供的代码,我发现了以下几个可能的问题:
1. **布局文件中的按钮点击事件未正确绑定**:虽然在 `SignUpActivity` 中已经设置了按钮的点击监听器,但需要确保布局文件中的按钮 ID 和 Java 代码中的 ID 完全一致。
2. **布局文件中的按钮高度设置有误**:第三个 `EditText`(用于再次输入密码)的高度被设置为 `match_parent`,这可能会导致按钮被挤压到屏幕外,从而无法点击。
3. **主活动启动了注册活动**:`MainActivity` 中直接启动了 `SignUpActivity`,这可能导致一些生命周期管理上的问题,但这不是主要问题,可以忽略。
4. **日志输出不完整**:为了更好地调试,可以在关键位置增加更多的日志输出。
### 解决方案
#### 1. 修改布局文件 `activity_sign_up.xml`
将第三个 `EditText` 的高度从 `match_parent` 改为 `wrap_content`,并确保所有按钮的 ID 正确。
```xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:background="@drawable/zhuce">
<include layout="@layout/main_titlr_bar"/>
<ImageView
android:id="@+id/LogoImage"
android:layout_width="match_parent"
android:layout_height="120dp"
android:layout_marginTop="50dp"
android:src="@drawable/zhucetouxiang" />
<TextView
android:id="@+id/TitleText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="0dp"
android:text="注册"
android:gravity="center"
android:textStyle="italic"
android:textColor="#fffef3"
android:textSize="30dp" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<EditText
android:id="@+id/UserNameEdit"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="15dp"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp"
android:layout_marginTop="10dp"
android:layout_marginBottom="10dp"
android:background="@drawable/translucent_edit"
android:hint="输入用户名"
android:textSize="24dp"
android:singleLine="true" />
<EditText
android:id="@+id/PassWordEdit"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="15dp"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp"
android:layout_marginTop="10dp"
android:layout_marginBottom="10dp"
android:background="@drawable/translucent_edit"
android:hint="输入密码"
android:textSize="24dp"
android:maxLength="16"
android:singleLine="true"
android:inputType="textPassword" />
<EditText
android:id="@+id/PassWordAgainEdit"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="15dp"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp"
android:layout_marginTop="10dp"
android:layout_marginBottom="10dp"
android:background="@drawable/translucent_edit"
android:hint="再次输入密码"
android:maxLength="16"
android:textSize="24dp"
android:singleLine="true"
android:inputType="textPassword" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/SignUpButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="15dp"
android:layout_margin="15dp"
android:layout_weight="1"
android:background="@drawable/translucent_button"
android:text="立即注册"
android:textColor="@color/white"
android:textSize="24dp" />
<Button
android:id="@+id/BackLoginButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="15dp"
android:layout_margin="15dp"
android:layout_weight="1"
android:textColor="@color/white"
android:background="@drawable/translucent_button"
android:text="返回登录"
android:textSize="24dp" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
```
#### 2. 修改 `SignUpActivity.java`
确保所有的按钮点击事件都能正常触发,并增加更多的日志输出以便调试。
```java
package com.example.englishears.activity;
import android.content.Intent;
import android.graphics.Color;
import android.os.Bundle;
import android.text.TextUtils;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import com.example.englishears.R;
import androidx.activity.EdgeToEdge;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.graphics.Insets;
import androidx.core.view.ViewCompat;
import androidx.core.view.WindowInsetsCompat;
public class SignUpActivity extends AppCompatActivity {
private TextView tvTitleText; // 标题
private Button btnSignUpButton, btnBackLoginButton; // 按钮
private EditText etUserName, etPassWord, etPassWordAgain; // 输入框
private String userName, passWord, passWordAgain; // 变量名改为小写以保持一致
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EdgeToEdge.enable(this);
setContentView(R.layout.activity_sign_up);
init();
ViewCompat.setOnApplyWindowInsetsListener(findViewById(R.id.main), (v, insets) -> {
Insets systemBars = insets.getInsets(WindowInsetsCompat.Type.systemBars());
v.setPadding(systemBars.left, systemBars.top, systemBars.right, systemBars.bottom);
return insets;
});
}
private void init() {
Log.d("SignUpActivity", "Initializing SignUpActivity");
tvTitleText = findViewById(R.id.TitleText);
btnSignUpButton = findViewById(R.id.SignUpButton);
btnBackLoginButton = findViewById(R.id.BackLoginButton);
etUserName = findViewById(R.id.UserNameEdit);
etPassWord = findViewById(R.id.PassWordEdit);
etPassWordAgain = findViewById(R.id.PassWordAgainEdit);
btnBackLoginButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Log.d("SignUpActivity", "Back button clicked");
finish();
}
});
btnSignUpButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Log.d("SignUpActivity", "Sign up button clicked");
getEditString();
if (TextUtils.isEmpty(userName)) {
Log.d("SignUpActivity", "Username is empty");
Toast.makeText(SignUpActivity.this, "请输入用户名", Toast.LENGTH_SHORT).show();
etUserName.setError("请输入用户名"); // 设置错误信息
return;
} else if (TextUtils.isEmpty(passWord)) {
Log.d("SignUpActivity", "Password is empty");
Toast.makeText(SignUpActivity.this, "请输入密码", Toast.LENGTH_SHORT).show();
etPassWord.setError("请输入密码"); // 设置错误信息
return;
} else if (TextUtils.isEmpty(passWordAgain)) {
Log.d("SignUpActivity", "Password again is empty");
Toast.makeText(SignUpActivity.this, "请再次输入密码", Toast.LENGTH_SHORT).show();
etPassWordAgain.setError("请再次输入密码"); // 设置错误信息
return;
} else if (!passWord.equals(passWordAgain)) {
Log.d("SignUpActivity", "Passwords do not match");
Toast.makeText(SignUpActivity.this, "输入两次的密码不一致", Toast.LENGTH_SHORT).show();
etPassWordAgain.setError("密码不一致"); // 设置错误信息
return;
} else {
Log.d("SignUpActivity", "Registration successful");
Toast.makeText(SignUpActivity.this, "注册成功", Toast.LENGTH_SHORT).show();
// 把用户名和密码保存到 SharedPreferences 文件中
// UtilsHelper.saveUserInfo(SignUpActivity.this, userName, passWord);
// 注册成功后把用户名传递到 LoginActivity 中
// Intent data = new Intent();
// data.putExtra("userName", userName);
// setResult(RESULT_OK, data);
finish();
}
}
});
}
private void getEditString() {
userName = etUserName.getText().toString().trim();
passWord = etPassWord.getText().toString().trim();
passWordAgain = etPassWordAgain.getText().toString().trim();
}
}
```
### 总结
通过上述修改,您应该能够解决按钮点击无反应的问题。如果仍有问题,请确保所有资源文件(如 `@drawable/zhuce`、`@drawable/translucent_edit` 等)都存在且路径正确。希望这些修改能帮助您顺利解决问题!
阅读全文
相关推荐
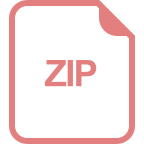
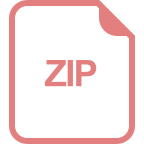
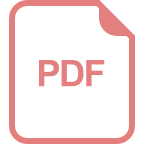







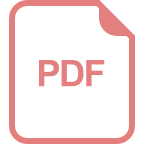
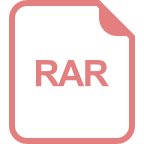
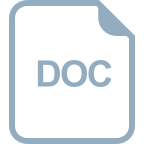





