用VScod创建一幅大小为240x320的图像,图像中心是一个大小为100x100的红色正方形,周围都是黑色
时间: 2024-09-23 21:13:50 浏览: 25
在VS Code中创建一个简单的图像并指定特定内容并不直接内置在代码编辑器的功能里,因为VS Code本质上是一个代码编辑器,而非图形设计或绘图工具。不过,你可以通过一些库,如P5.js(如果是在JavaScript环境中)或Python Imaging Library (PIL)(如果是在Python环境下)来实现这个需求。
这里以Python和PIL为例,展示如何做:
```python
from PIL import Image, ImageDraw
# 创建一个新的240x320像素的白色背景图像
img = Image.new('RGB', (240, 320), color='white')
# 使用ImageDraw模块绘制一个黑色边框
draw = ImageDraw.Draw(img)
draw.rectangle([(120 - 50, 160 - 50), (120 + 50, 160 + 50)], fill='black') # 左上角坐标减去半边长,右下角加上半边长
# 在图像中心画一个红色100x100的正方形
draw.rectangle([(120 - 50, 160 - 50), (120 + 50, 160 + 50)], outline='red', width=0)
# 保存图像
img.save('image_with_square.png')
```
这将生成一个名为`image_with_square.png`的文件,显示了你所描述的图案。
相关问题
创建一幅大小为240x320的图像,图像中心是一个大小为100x100的红色正方形,周围都是黑色
创建一幅大小为240x320像素的图像,你可以使用Python的PIL(Pillow)库来实现。首先,我们需要导入必要的模块并初始化一个新的Image对象。然后,我们将在这个新的白色背景上绘制一个红色的正方形,使其位于图像的中心位置。
以下是步骤和代码示例:
```python
from PIL import Image, ImageDraw, ImageFont
# 初始化一个240x320的黑底白字图片
img = Image.new('RGB', (240, 320), color='black')
draw = ImageDraw.Draw(img)
# 计算并设置红色正方形的左上角坐标,保证它位于图片中心
width, height = img.size
square_width, square_height = 100, 100
center_x = width // 2 - square_width // 2
center_y = height // 2 - square_height // 2
# 绘制红色正方形
draw.rectangle([(center_x, center_y), (center_x + square_width, center_y + square_height)], fill='red')
# 显示或保存图像
img.save("center_square.png")
```
创建一幅大小为240x320的图像,图像中心是一个大小为100x100的红色正方形,周围是黑
色边框,图像保存为test.png文件,代码如下:
```python
from PIL import Image, ImageDraw
# 创建一幅大小为240x320的图像
img = Image.new('RGB', (240, 320), color='black')
# 在图像中心创建一个大小为100x100的红色正方形
draw = ImageDraw.Draw(img)
square_size = 100
square_center = (img.width // 2, img.height // 2)
square_top_left = (square_center[0] - square_size // 2, square_center[1] - square_size // 2)
square_bottom_right = (square_center[0] + square_size // 2, square_center[1] + square_size // 2)
draw.rectangle([square_top_left, square_bottom_right], fill='red')
# 在正方形周围创建黑色边框
border_size = 5
border_top_left = (square_top_left[0] - border_size, square_top_left[1] - border_size)
border_bottom_right = (square_bottom_right[0] + border_size, square_bottom_right[1] + border_size)
draw.rectangle([border_top_left, border_bottom_right], outline='black', width=border_size)
# 保存图像为test.png文件
img.save('test.png')
```
这段代码使用了Pillow库,首先创建了一幅大小为240x320的黑色图像,然后在图像中心创建了一个大小为100x100的红色正方形,最后在正方形周围创建了一个黑色边框,并将图像保存为test.png文件。
相关推荐
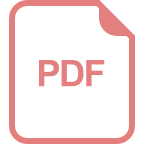
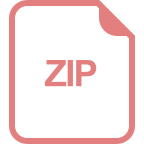













