java极大极小值算法实现五子棋
时间: 2024-05-09 12:11:29 浏览: 25
在Java中实现五子棋的极大极小算法,可以采用博弈树搜索的方式来进行。下面是一个简单的实现示例:
```java
public class Gomoku {
private static final int SIZE = 15; // 棋盘大小
private static final int EMPTY = 0; // 空位置
private static final int BLACK = 1; // 黑棋
private static final int WHITE = 2; // 白棋
private int[][] board; // 棋盘
public Gomoku() {
board = new int[SIZE][SIZE];
}
// 极大极小值算法
public int[] minimax(int depth, int player) {
int[] result = new int[3]; // 结果数组,包含最佳位置和对应的分数
result[2] = (player == BLACK) ? Integer.MIN_VALUE : Integer.MAX_VALUE;
if (depth == 0 || isGameOver()) {
result[2] = evaluate();
return result;
}
List<int[]> moves = generateMoves();
for (int[] move : moves) {
int row = move[0];
int col = move[1];
board[row][col] = player;
if (player == BLACK) {
int[] currentResult = minimax(depth - 1, WHITE);
if (currentResult[2] > result[2]) {
result[0] = row;
result[1] = col;
result[2] = currentResult[2];
}
} else {
int[] currentResult = minimax(depth - 1, BLACK);
if (currentResult[2] < result[2]) {
result[0] = row;
result[1] = col;
result[2] = currentResult[2];
}
}
board[row][col] = EMPTY; // 撤销当前落子
}
return result;
}
// 生成所有可能的落子位置
private List<int[]> generateMoves() {
List<int[]> moves = new ArrayList<>();
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
if (board[i][j] == EMPTY) {
moves.add(new int[]{i, j});
}
}
}
return moves;
}
// 评估当前局面得分
private int evaluate() {
// 实现自己的评估函数,根据棋盘状态给出一个分数
return 0;
}
// 判断游戏是否结束
private boolean isGameOver() {
// 实现自己的游戏结束判断逻辑
return false;
}
}
```
以上是一个简单的极大极小值算法实现的五子棋示例,其中`minimax`方法使用递归实现了极大极小值搜索,`generateMoves`方法生成所有可能的落子位置,`evaluate`方法评估当前局面得分,`isGameOver`方法判断游戏是否结束。
相关推荐
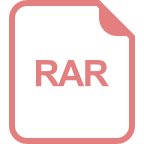














