完成加减乘除四则运算自定义函数编写作业修改,使用表单提交运算数据
时间: 2024-12-12 08:21:47 浏览: 3
当你需要编写一个接受用户输入并进行加、减、乘、除四则运算的自定义函数时,你可以按照以下步骤进行:
1. **设置HTML表单**:
创建一个简单的HTML表单,让用户输入两个数字以及选择运算符(加、减、乘、除)。这通常包含`<input>`元素用于数字输入,`<select>`元素用于运算符选择。
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>四则运算</title>
</head>
<body>
<form id="calculator-form" onsubmit="return performCalculation(event)">
<label for="num1">Number 1:</label>
<input type="number" id="num1" required><br>
<label for="num2">Number 2:</label>
<input type="number" id="num2" required><br>
<label for="operator">Operator:</label>
<select id="operator" required>
<option value="+">+</option>
<option value="-">-</option>
<option value="*">*</option>
<option value="/">/</option>
</select><br>
<button type="submit">Calculate</button>
</form>
<script src="your-python-function.js"></script>
</body>
</html>
```
2. **JavaScript处理表单提交**:
在`performCalculation`函数中,阻止表单默认提交行为,然后获取用户输入的值,调用Python函数进行计算并将结果显示给用户。由于浏览器环境不能直接执行Python代码,你需要提供一个接口来调用后端服务器上的Python脚本,例如使用`fetch`或`axios`等库发送请求。
```javascript
function performCalculation(event) {
event.preventDefault(); // 阻止表单自动提交
const num1 = document.getElementById('num1').value;
const num2 = document.getElementById('num2').value;
const operator = document.getElementById('operator').value;
fetch('/calculate', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ num1, num2, operator }),
})
.then(response => response.json())
.then(result => displayResult(result))
.catch(error => console.error('Error:', error));
}
function displayResult(result) {
// 将结果显示在一个合适的容器里,比如`<p>`标签
document.getElementById('result').innerText = `Result: ${result}`;
}
```
3. **Python后端处理**:
在服务器端,如Flask或Django应用中,接收前端传来的请求,解析参数,执行相应的运算,然后返回结果。这里假设你已经有一个名为`app.py`的Flask应用:
```python
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/calculate', methods=['POST'])
def calculate():
data = request.get_json()
num1 = data['num1']
num2 = data['num2']
operator = data['operator']
if operator == '+':
result = num1 + num2
elif operator == '-':
result = num1 - num2
elif operator == '*':
result = num1 * num2
elif operator == '/':
try:
result = num1 / num2
except ZeroDivisionError:
return jsonify({'error': 'Cannot divide by zero'}), 400
return jsonify({'result': result})
if __name__ == '__main__':
app.run(debug=True)
```
确保你的Python服务运行起来并监听指定的端口,例如`http://localhost:5000`。
阅读全文
相关推荐
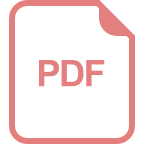
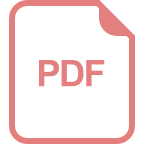
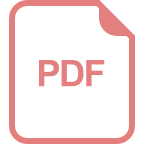














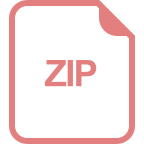
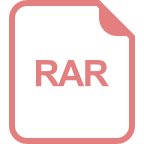