c语言浮点数四舍五入
时间: 2023-12-02 15:39:10 浏览: 45
在C语言中,浮点数四舍五入可以通过使用数学库函数round()来实现。round()函数的作用是将一个浮点数四舍五入为最接近的整数。如果浮点数的小数部分大于或等于0.5,则将其向上舍入到最接近的整数;否则,将其向下舍入到最接近的整数。以下是一个使用round()函数进行四舍五入的例子:
#include <stdio.h>
#include <math.h>
int main()
{
float num = 3.14159;
int rounded_num = round(num);
printf("原始数值:%f\n", num);
printf("四舍五入后的整数:%d\n", rounded_num);
return 0;
}
输出结果为:
原始数值:3.141590
四舍五入后的整数:3
需要注意的是,round()函数需要包含头文件<math.h>。同时,如果需要保留小数位数,可以使用printf()函数的格式化输出来实现。例如,使用“%.2f”可以保留两位小数。
相关问题
C语言浮点数四舍五入
C语言中浮点数的四舍五入可以使用round函数实现。round函数的原型为double round(double x),其返回值为x的四舍五入值。需要注意的是,round函数是向最近的整数舍入,如果距离两个整数的距离相等,则向偶数舍入。例如,round(0.5)的返回值为0,而round(1.5)的返回值为2。
以下是一个C语言浮点数四舍五入的例子:
```c
#include <stdio.h>
#include <math.h>
int main()
{
double x = 3.14159;
double y = -2.71828;
double z = 1.5;
double w = 2.5;
printf("round(%f) = %f\n", x, round(x)); // 输出:3.000000
printf("round(%f) = %f\n", y, round(y)); // 输出:-3.000000
printf("round(%f) = %f\n", z, round(z)); // 输出:2.000000
printf("round(%f) = %f\n", w, round(w)); // 输出:2.000000
return 0;
}
```
c语言 浮点数四舍五入
在C语言中,我们可以通过两种方法来实现浮点数的四舍五入。
方法一:利用取整规则(不使用库函数)。可以将浮点数乘以一个指定的倍数,然后将结果转换为整数,再除以倍数得到四舍五入后的结果。比如,如果要将一个浮点数保留两位小数并四舍五入,可以将其乘以100,转换为整数后再除以100.0。这样就可以实现四舍五入的效果。
方法二:使用C语言提供的库函数round函数。这个函数可以将一个浮点数四舍五入为最接近的整数。要使用round函数,需要在程序中引用"math.h"头文件,并将需要四舍五入的浮点数作为参数传入round函数。round函数会返回一个整数,表示四舍五入后的结果。
下面是使用round函数实现浮点数四舍五入的示例代码:
```c
#include <stdio.h>
#include <math.h>
int main() {
float num = 3.14159;
num = round(num * 10000) / 10000.0;
printf("%.4f", num);
}
```
以上代码将保留3.14159的四位小数,并进行四舍五入后输出。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [四舍五入(C语言实现)](https://blog.csdn.net/m0_72482689/article/details/125997219)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *3* [python中实现精确的浮点数运算详解](https://download.csdn.net/download/weixin_38718434/13764793)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
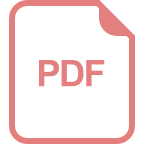
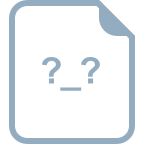












