用Python写一段代码,内容是设计一个温度换算器,实现华氏度,摄氏度,开氏度之间的相互转换。其中,摄氏度=(华氏度-32)/1.8;华氏度=摄氏度*1.8+32;开氏度=摄氏度+273.15.并运行一下这一段代码
时间: 2024-10-18 15:19:35 浏览: 53
当然可以,下面是一个简单的Python程序,用于实现华氏度、摄氏度和开氏度之间的转换:
```python
class TemperatureConverter:
def celsius_to_fahrenheit(self, celsius):
return (celsius * 1.8) + 32
def fahrenheit_to_celsius(self, fahrenheit):
return (fahrenheit - 32) / 1.8
def celsius_to_kelvin(self, celsius):
return celsius + 273.15
def kelvin_to_celsius(self, kelvin):
return kelvin - 273.15
# 示例使用
def main():
print("请输入你要转换的温度单位及数值:")
unit = input("请选择转换类型(F -> 华氏度,C -> 摄氏度,K -> 开氏度):")
value = float(input(f"{unit}度值: "))
if unit.upper() == 'F':
converted_value = TemperatureConverter().celsius_to_fahrenheit(value)
print(f"{value}华氏度等于{converted_value:.2f}摄氏度")
elif unit.upper() == 'C':
converted_value = TemperatureConverter().celsius_to_fahrenheit(value)
print(f"{value}摄氏度等于{converted_value:.2f}华氏度")
converted_value = TemperatureConverter().celsius_to_kelvin(value)
print(f"{value}摄氏度等于{converted_value:.2f}开氏度")
elif unit.upper() == 'K':
converted_value = TemperatureConverter().kelvin_to_celsius(value)
print(f"{value}开氏度等于{converted_value:.2f}摄氏度")
else:
print("输入的单位无效,请选择正确的选项。")
if __name__ == "__main__":
main()
```
当你运行这段代码时,它会提示用户输入他们想要转换的温度类型(华氏度、摄氏度或开氏度)以及对应的值,然后进行相应的转换。
阅读全文
相关推荐
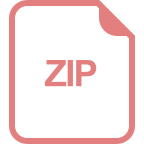
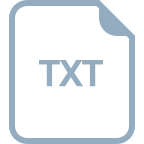
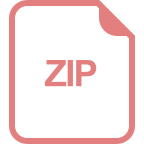













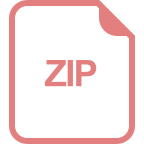

