如何用Python编写代码来实现摄氏度到华氏度的转换以及反过来的华氏度到摄氏度的换算?
时间: 2024-09-25 18:12:57 浏览: 39
在Python中,你可以创建函数分别用于摄氏度转华氏度和华氏度转摄氏度的计算。以下是简单的实现:
```python
# 摄氏度转华氏度
def celsius_to_fahrenheit(celsius):
fahrenheit = (celsius * 9/5) + 32
return fahrenheit
# 华氏度转摄氏度
def fahrenheit_to_celsius(fahrenheit):
celsius = (fahrenheit - 32) * 5/9
return celsius
# 示例
celsius_temp = 20
print("20摄氏度等于", celsius_to_fahrenheit(celsius_temp), "华氏度")
fahrenheit_temp = 68
print("68华氏度等于", fahrenheit_to_celsius(fahrenheit_temp), "摄氏度")
```
使用这两个函数,你可以轻松地在两种温度单位之间切换。如果你想要连续转换,例如从摄氏度开始并自动转换回摄氏度,只需在函数之间交替调用即可。
相关问题
编写一个Python程序,实现摄氏度到华氏度的转换
当然可以。在Python中,你可以创建一个简单的函数来实现摄氏度转华氏度的计算。摄氏温度和华氏温度之间的转换公式是:\( F = C \times 1.8 + 32 \),其中\( F \)代表华氏温度,\( C \)代表摄氏温度。下面是一个示例程序:
```python
def celsius_to_fahrenheit(celsius):
fahrenheit = celsius * 1.8 + 32
return fahrenheit
# 示例:将25摄氏度转换为华氏度
celsius_input = 25
fahrenheit_output = celsius_to_fahrenheit(celsius_input)
print(f"{celsius_input}摄氏度等于{fahrenheit_output}华氏度.")
# 如果你想让用户输入摄氏度并得到相应的华氏度,可以这样:
celsius_user_input = float(input("请输入摄氏温度: "))
fahrenheit_user_output = celsius_to_fahrenheit(celsius_user_input)
print(f"{celsius_user_input}摄氏度等于{fahrenheit_user_output}华氏度.")
```
在这个例子中,我们首先定义了一个名为`celsius_to_fahrenheit`的函数,然后进行了一个具体的温度转换。如果你想让用户输入,只需添加一个`input`函数获取用户输入,并将其转换为浮点数。
用Python写出摄氏度与华氏度的转换用Python写出摄氏度与华氏度的转换
好的,以下是Python程序示例,用于将摄氏度转换为华氏度和将华氏度转换为摄氏度:
```python
# 将摄氏度转换为华氏度
def celsius_to_fahrenheit(celsius):
fahrenheit = (celsius * 1.8) + 32
return fahrenheit
# 将华氏度转换为摄氏度
def fahrenheit_to_celsius(fahrenheit):
celsius = (fahrenheit - 32) / 1.8
return celsius
# 测试
celsius = 28 # 摄氏度
fahrenheit = 82.4 # 华氏度
print("{}摄氏度 = {}华氏度".format(celsius, celsius_to_fahrenheit(celsius)))
print("{}华氏度 = {}摄氏度".format(fahrenheit, fahrenheit_to_celsius(fahrenheit)))
```
在上述代码中,我们定义了两个函数,`celsius_to_fahrenheit()` 和 `fahrenheit_to_celsius()`,分别用于将摄氏度转换为华氏度和将华氏度转换为摄氏度。
在 `celsius_to_fahrenheit()` 函数中,我们使用公式 `fahrenheit = (celsius * 1.8) + 32` 将摄氏度转换为华氏度,并返回转换后的值。
在 `fahrenheit_to_celsius()` 函数中,我们使用公式 `celsius = (fahrenheit - 32) / 1.8` 将华氏度转换为摄氏度,并返回转换后的值。
接着,我们定义了两个变量 `celsius` 和 `fahrenheit`,分别代表摄氏度和华氏度,并输出将其转换后的值。
需要注意的是,这里我们使用了字符串格式化输出的方式,使用 `{}` 占位符来表示需要填充的值,然后使用 `format()` 函数将变量的值填充到占位符中。
阅读全文
相关推荐
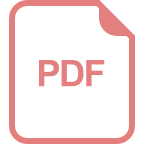
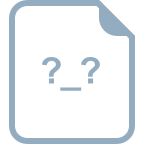
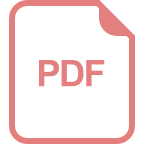













