numpy 汉明距离
时间: 2023-10-09 22:06:33 浏览: 149
汉明距离是衡量两个等长字符串之间的差异度量。在NumPy中,可以使用以下方法计算两个数组的汉明距离:
```python
import numpy as np
def hamming_distance(arr1, arr2):
# 确保两个数组具有相同的形状
assert arr1.shape == arr2.shape, "Arrays must have the same shape"
# 比较两个数组的元素是否相等,返回一个布尔数组
equal_elements = (arr1 != arr2)
# 统计布尔数组中True的个数,即为汉明距离
distance = np.sum(equal_elements)
return distance
```
这个函数接受两个NumPy数组作为输入,并返回它们之间的汉明距离。请注意,这个函数要求输入的两个数组具有相同的形状。你可以将自己的数组传递给这个函数来计算汉明距离,例如:
```python
arr1 = np.array([0, 1, 0, 1, 0])
arr2 = np.array([1, 1, 0, 0, 1])
distance = hamming_distance(arr1, arr2)
print(distance) # 输出:3
```
在上面的例子中,`arr1`和`arr2`是具有相同形状的NumPy数组,汉明距离为3。
相关问题
用汉明距离公式计算S盒的非线性度代码
以下是一个用汉明距离公式计算S盒的非线性度的示例代码(使用Python语言):
```python
import numpy as np
# 定义S盒的真值表
S_BOX = np.array([
[0x9, 0x4, 0xa, 0xb, 0xd, 0x1, 0x8, 0x5, 0x6, 0x2, 0x0, 0x3, 0xc, 0xe, 0xf, 0x7],
[0x0, 0xf, 0xa, 0xe, 0x6, 0xb, 0x4, 0x2, 0xd, 0x1, 0xc, 0x7, 0x8, 0x5, 0x9, 0x3],
[0xe, 0xb, 0x4, 0xc, 0x6, 0xd, 0xf, 0xa, 0x2, 0x3, 0x8, 0x1, 0x0, 0x7, 0x5, 0x9],
[0x5, 0x8, 0x1, 0xd, 0xa, 0x3, 0x4, 0x2, 0xe, 0xf, 0xc, 0x7, 0x6, 0x0, 0x9, 0xb],
[0x7, 0xd, 0xa, 0x1, 0x0, 0x8, 0x9, 0xf, 0xe, 0x4, 0x6, 0xc, 0xb, 0x2, 0x5, 0x3],
[0xc, 0x1, 0x6, 0xd, 0xf, 0x0, 0x9, 0xa, 0x4, 0x5, 0xb, 0x7, 0x2, 0x8, 0x3, 0xe],
[0x2, 0xc, 0x4, 0x1, 0x7, 0xa, 0xb, 0x6, 0x8, 0x5, 0x3, 0xf, 0xd, 0x0, 0xe, 0x9],
[0xe, 0x8, 0x2, 0x5, 0x6, 0x9, 0x1, 0xc, 0xf, 0x4, 0xb, 0x0, 0xd, 0xa, 0x3, 0x7]
])
# 计算S盒的非线性度
def calculate_nonlinearity(s_box):
n = len(s_box[0])
max_delta_y = 0
for y in range(n):
# 计算输出比特y在S盒输出中的出现次数
output_count = [0] * (n // 2)
for x in range(n):
output_count[s_box[x] ^ s_box[x ^ y]] += 1
# 计算输出比特y与其他输出比特之间的汉明距离
delta_y = []
for i in range(n):
if i != y:
temp = 0
for j in range(n // 2):
if output_count[j] != 0:
temp += bin(j ^ (s_box[i] ^ s_box[i ^ y])).count('1') * output_count[j]
delta_y.append(temp)
# 更新最大汉明距离
if delta_y:
max_delta_y = max(max_delta_y, max(delta_y))
# 计算S盒的非线性度
nonlinearity = (2 ** (n - 1)) - (0.5 * max_delta_y)
return nonlinearity
# 打印S盒的非线性度
print("S盒的非线性度为:", calculate_nonlinearity(S_BOX))
```
该代码可以计算任意S盒的非线性度,并可根据需要进行修改。
随机选择/下载一个数据集,或生成一些数据 1.绘制盒装图、柱状图、散点图、折线图等 2.编写常用距离和相似度计算程序 闵可夫斯基距离 曼哈顿距离 欧式距离 切比雪夫距离 余弦相似度 Jaccard 相似度 汉明距离
随机选择或下载数据集通常可以在很多在线资源上完成,例如Kaggle、UCI Machine Learning Repository或者是GitHub上的各种数据仓库。在Python中,你可以使用pandas库加载CSV文件,比如`pd.read_csv('data.csv')`。
绘制图表方面,Python的matplotlib库可以绘制各种图形,如`plt.boxplot(data)`, `plt.bar(x, heights)`等。Scikit-learn库中的`pairwise_distances()`函数能帮助计算多种距离,如:
```python
from sklearn.metrics.pairwise import euclidean_distances, manhattan_distances, chebyshev_distance
import numpy as np
# 示例数据
data = np.random.rand(10, 2)
# 欧式距离
euclid_dist = euclidean_distances(data)
# 曼哈顿距离
manhat_dist = manhattan_distances(data)
# 切比雪夫距离
cheby_dist = chebyshev_distance(data)
for dist_name in ['euclidean', 'manhattan', 'chebyshev']:
print(f"{dist_name} distance matrix:\n{getattr(distances, dist_name)(data)}")
计算相似度,例如余弦相似度,可以使用TfidfVectorizer或CountVectorizer配合scipy的cosine_similarity:
```python
from sklearn.feature_extraction.text import TfidfVectorizer
from scipy.spatial.distance import cosine
vectorizer = TfidfVectorizer()
tfidf_matrix = vectorizer.fit_transform(corpus)
similarity_scores = 1 - cosine(tfidf_matrix[0], tfidf_matrix[1])
```
对于Jaccard相似度和汉明距离,它们一般用于集合和序列的比较,可以使用`sklearn.feature_extraction.text`中的`jaccard_score()`和`hamming_loss()`函数:
```python
from sklearn.metrics.cluster import jaccard_score, adjusted_rand_score
def calculate_jaccard(a, b):
return jaccard_score([a], [b])
# 使用类似方法计算其他相似度和损失
```
阅读全文
相关推荐
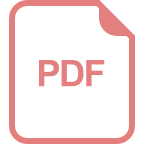
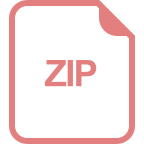
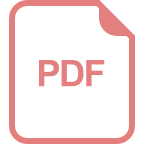
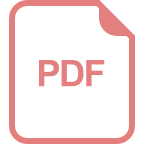
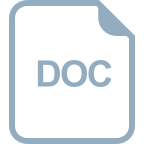
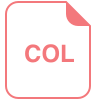
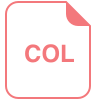




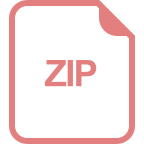