写出RSA算法的c语言代码
时间: 2025-01-04 09:21:45 浏览: 8
RSA算法是一种非对称加密技术,它由Ron Rivest、Adi Shamir和Leonard Adleman于1977年首次提出。以下是一个简单的RSA算法在C语言中的基础实现,主要包括生成密钥对、加密和解密过程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义大素数的长度
#define PRIME_SIZE 512
// 算法中的辅助函数
void gcd(int a, int b, int *x, int *y);
int isPrime(int num);
// RSA关键操作
void generateKeys();
void encrypt(char *plaintext, char **ciphertext);
void decrypt(char *ciphertext, char *plaintext);
int main() {
// 初始化并生成密钥对
generateKeys();
// 示例:加密和解密
char message[] = "Hello, RSA!";
encrypt(message, &ciphertext); // ciphertext will be stored in an external buffer
printf("Encrypted message: %s\n", ciphertext);
decrypt(ciphertext, plaintext); // plaintext will be updated in place
printf("Decrypted message: %s\n", plaintext);
return 0;
}
// RSA核心部分:生成公钥和私钥
void generateKeys() {
// ... 实现大素数生成、模数计算等步骤 ...
}
// GCD函数用于计算最大公约数
void gcd(int a, int b, int *x, int *y) {
// ... 实现欧几里得算法 ...
}
// 检查是否为质数
int isPrime(int num) {
// ... 实现质数判断 ...
}
// 加密函数
void encrypt(char *plaintext, char **ciphertext) {
// ... 使用模指数运算 ...
}
// 解密函数
void decrypt(char *ciphertext, char *plaintext) {
// ... 使用私钥和模逆运算 ...
}
```
请注意,这只是一个非常简化的示例,实际的RSA实现会包含更复杂的数学运算和错误处理,并且通常不会在这里全部展示。在实际应用中,你需要将上述的部分替换为完整的质数搜索、密钥生成、以及加解密过程的细节。
阅读全文
相关推荐




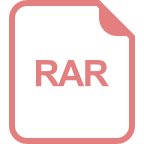

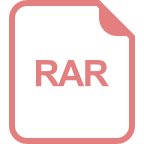












