用c语言编程:利用结构体,输入学生学号、数学成绩、语文成绩、英语成绩、姓名,按学号顺序输出该学生学号、姓名和三门科目成绩的平均分
时间: 2024-09-19 13:10:22 浏览: 52
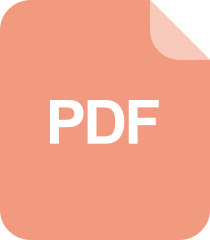
C语言利用结构体数组实现学生成绩管理系统

在C语言中,你可以创建一个结构体来存储学生的相关信息,并结合数组或者动态内存分配来完成这个任务。首先,定义一个名为`Student`的结构体:
```c
struct Student {
int id; // 学号
float mathScore; // 数学成绩
float chineseScore; // 语文成绩
float englishScore; // 英语成绩
char name[50]; // 姓名
};
```
然后编写一个函数,用于读取用户输入并计算平均分:
```c
void printStudentAverage(struct Student students[], int numStudents) {
for (int i = 0; i < numStudents; i++) {
float totalScore = students[i].mathScore + students[i].chineseScore + students[i].englishScore;
float average = totalScore / 3.0;
printf("学号: %d, 姓名: %s, 平均分: %.2f\n", students[i].id, students[i].name, average);
}
}
```
接下来,你需要提供一个主函数,接收用户的输入,创建结构体数组,并调用上述打印函数:
```c
int main() {
int numStudents;
printf("请输入学生人数: ");
scanf("%d", &numStudents);
struct Student* studentList = malloc(numStudents * sizeof(struct Student)); // 动态分配内存
for (int i = 0; i < numStudents; i++) {
printf("请输入第%d位同学的信息:\n", i+1);
scanf("%d", &studentList[i].id);
scanf("%f", &studentList[i].mathScore);
scanf("%f", &studentList[i].chineseScore);
scanf("%f", &studentList[i].englishScore);
getchar(); // 后跟getchar()以清除换行符对scanf的影响
fgets(studentList[i].name, sizeof(studentList[i].name), stdin); // 读取字符串
studentList[i].name[strlen(studentList[i].name)-1] = '\0'; // 去除字符串末尾的'\n'
}
printStudentAverage(studentList, numStudents);
free(studentList); // 释放动态分配的内存
return 0;
}
```
阅读全文
相关推荐
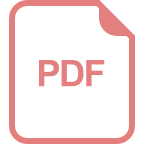
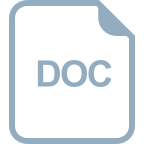



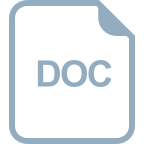

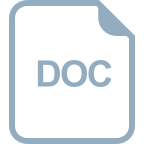
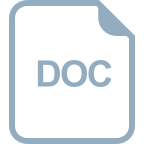


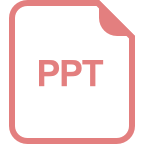
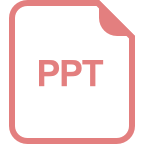




