填充刚刚的代码主要是矩阵乘法
时间: 2024-06-23 15:02:25 浏览: 7
在计算机编程中,矩阵乘法是一项基本的线性代数操作,特别是在处理多变量方程组、图像处理和机器学习等领域非常常见。填充代码通常是为了解决矩阵乘法的问题,这通常涉及到实现两个矩阵相乘的过程。
矩阵乘法(也称为矩阵积)规则是:第一个矩阵的列数必须等于第二个矩阵的行数,结果矩阵的元素是将第一个矩阵的行元素与第二个矩阵对应列元素对应相乘后求和得到的。例如,假设我们有两个矩阵A和B,A的维度为m×n,B的维度为n×p,那么它们的乘积C的维度将是m×p,其中C[i][j] = Σ(A[i][k] * B[k][j]),这里Σ表示求和,k是遍历的中间索引。
填充代码的具体实现可能取决于编程语言和库的选择。如果是使用像Python的NumPy库,可以用一行简洁的代码`result = np.dot(matrix1, matrix2)`完成。如果是手动编写,可能会涉及到嵌套循环,遍历矩阵中的每个元素执行乘法和累加操作。
相关问题
c语言实现矩阵乘法的循环展开
矩阵乘法的循环展开是一种优化技术,可以提高矩阵乘法的性能。下面是一个使用C语言实现矩阵乘法的循环展开的示例代码:
```c
#include <stdio.h>
#define N 1000
void matrix_multiply(int A[N][N], int B[N][N], int C[N][N])
{
for (int i = 0; i < N; i++)
{
for (int j = 0; j < N; j++)
{
C[i][j] = 0;
for (int k = 0; k < N; k += 4)
{
C[i][j] += A[i][k] * B[k][j];
C[i][j] += A[i][k+1] * B[k+1][j];
C[i][j] += A[i][k+2] * B[k+2][j];
C[i][j] += A[i][k+3] * B[k+3][j];
}
}
}
}
int main()
{
int A[N][N];
int B[N][N];
int C[N][N];
// 填充A和B矩阵,这里省略填充过程
matrix_multiply(A, B, C);
// 打印结果矩阵C,这里省略打印过程
return 0;
}
```
在上面的示例代码中,我们使用了一个步长为4的循环展开,每次同时计算四个元素的乘积并累加到结果矩阵C中。这样可以减少循环迭代次数,提高计算效率。你可以根据实际需求调整步长大小,但需要确保矩阵的大小是步长的倍数。
用C++编写strassen矩阵乘法分治法
以下是使用C语言编写的Strassen矩阵乘法分治法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
void matrix_add(int n, int **a, int **b, int **c) {
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
c[i][j] = a[i][j] + b[i][j];
}
}
}
void matrix_sub(int n, int **a, int **b, int **c) {
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
c[i][j] = a[i][j] - b[i][j];
}
}
}
void matrix_multiply_simple(int n, int **a, int **b, int **c) {
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
c[i][j] = 0;
for (int k = 0; k < n; k++) {
c[i][j] += a[i][k] * b[k][j];
}
}
}
}
void matrix_multiply_strassen(int n, int **a, int **b, int **c) {
if (n <= 64) { // 使用普通矩阵乘法
matrix_multiply_simple(n, a, b, c);
return;
}
int **a11 = (int **) malloc(n / 2 * sizeof(int *));
int **a12 = (int **) malloc(n / 2 * sizeof(int *));
int **a21 = (int **) malloc(n / 2 * sizeof(int *));
int **a22 = (int **) malloc(n / 2 * sizeof(int *));
int **b11 = (int **) malloc(n / 2 * sizeof(int *));
int **b12 = (int **) malloc(n / 2 * sizeof(int *));
int **b21 = (int **) malloc(n / 2 * sizeof(int *));
int **b22 = (int **) malloc(n / 2 * sizeof(int *));
int **c11 = (int **) malloc(n / 2 * sizeof(int *));
int **c12 = (int **) malloc(n / 2 * sizeof(int *));
int **c21 = (int **) malloc(n / 2 * sizeof(int *));
int **c22 = (int **) malloc(n / 2 * sizeof(int *));
int **m1 = (int **) malloc(n / 2 * sizeof(int *));
int **m2 = (int **) malloc(n / 2 * sizeof(int *));
int **m3 = (int **) malloc(n / 2 * sizeof(int *));
int **m4 = (int **) malloc(n / 2 * sizeof(int *));
int **m5 = (int **) malloc(n / 2 * sizeof(int *));
int **m6 = (int **) malloc(n / 2 * sizeof(int *));
int **m7 = (int **) malloc(n / 2 * sizeof(int *));
for (int i = 0; i < n / 2; i++) {
a11[i] = (int *) malloc(n / 2 * sizeof(int));
a12[i] = (int *) malloc(n / 2 * sizeof(int));
a21[i] = (int *) malloc(n / 2 * sizeof(int));
a22[i] = (int *) malloc(n / 2 * sizeof(int));
b11[i] = (int *) malloc(n / 2 * sizeof(int));
b12[i] = (int *) malloc(n / 2 * sizeof(int));
b21[i] = (int *) malloc(n / 2 * sizeof(int));
b22[i] = (int *) malloc(n / 2 * sizeof(int));
c11[i] = (int *) malloc(n / 2 * sizeof(int));
c12[i] = (int *) malloc(n / 2 * sizeof(int));
c21[i] = (int *) malloc(n / 2 * sizeof(int));
c22[i] = (int *) malloc(n / 2 * sizeof(int));
m1[i] = (int *) malloc(n / 2 * sizeof(int));
m2[i] = (int *) malloc(n / 2 * sizeof(int));
m3[i] = (int *) malloc(n / 2 * sizeof(int));
m4[i] = (int *) malloc(n / 2 * sizeof(int));
m5[i] = (int *) malloc(n / 2 * sizeof(int));
m6[i] = (int *) malloc(n / 2 * sizeof(int));
m7[i] = (int *) malloc(n / 2 * sizeof(int));
}
// 将矩阵 a 和 b 拆分成四个子矩阵
for (int i = 0; i < n / 2; i++) {
for (int j = 0; j < n / 2; j++) {
a11[i][j] = a[i][j];
a12[i][j] = a[i][j + n / 2];
a21[i][j] = a[i + n / 2][j];
a22[i][j] = a[i + n / 2][j + n / 2];
b11[i][j] = b[i][j];
b12[i][j] = b[i][j + n / 2];
b21[i][j] = b[i + n / 2][j];
b22[i][j] = b[i + n / 2][j + n / 2];
}
}
// 计算七个子矩阵的乘积
matrix_add(n / 2, a11, a22, m1);
matrix_add(n / 2, b11, b22, m2);
matrix_multiply_strassen(n / 2, m1, m2, c11);
matrix_add(n / 2, a21, a22, m1);
matrix_multiply_strassen(n / 2, m1, b11, c21);
matrix_sub(n / 2, b12, b22, m1);
matrix_multiply_strassen(n / 2, a11, m1, c12);
matrix_sub(n / 2, b21, b11, m1);
matrix_multiply_strassen(n / 2, a22, m1, c22);
matrix_add(n / 2, a11, a12, m1);
matrix_multiply_strassen(n / 2, m1, b22, m2);
matrix_sub(n / 2, c11, c12, m3);
matrix_add(n / 2, c21, m2, m4);
matrix_add(n / 2, c12, m2, m5);
matrix_sub(n / 2, a21, a11, m1);
matrix_add(n / 2, b11, b12, m2);
matrix_multiply_strassen(n / 2, m1, m2, m6);
matrix_sub(n / 2, c22, m5, m7);
// 计算结果矩阵
matrix_add(n / 2, m3, m5, c11);
matrix_add(n / 2, m4, m6, c12);
matrix_add(n / 2, m1, m5, c21);
matrix_add(n / 2, m2, m7, c22);
for (int i = 0; i < n / 2; i++) {
for (int j = 0; j < n / 2; j++) {
c[i][j] = c11[i][j];
c[i][j + n / 2] = c12[i][j];
c[i + n / 2][j] = c21[i][j];
c[i + n / 2][j + n / 2] = c22[i][j];
}
}
// 释放内存
for (int i = 0; i < n / 2; i++) {
free(a11[i]);
free(a12[i]);
free(a21[i]);
free(a22[i]);
free(b11[i]);
free(b12[i]);
free(b21[i]);
free(b22[i]);
free(c11[i]);
free(c12[i]);
free(c21[i]);
free(c22[i]);
free(m1[i]);
free(m2[i]);
free(m3[i]);
free(m4[i]);
free(m5[i]);
free(m6[i]);
free(m7[i]);
}
free(a11);
free(a12);
free(a21);
free(a22);
free(b11);
free(b12);
free(b21);
free(b22);
free(c11);
free(c12);
free(c21);
free(c22);
free(m1);
free(m2);
free(m3);
free(m4);
free(m5);
free(m6);
free(m7);
}
int main() {
int n = 4;
int **a = (int **) malloc(n * sizeof(int *));
int **b = (int **) malloc(n * sizeof(int *));
int **c = (int **) malloc(n * sizeof(int *));
for (int i = 0; i < n; i++) {
a[i] = (int *) malloc(n * sizeof(int));
b[i] = (int *) malloc(n * sizeof(int));
c[i] = (int *) malloc(n * sizeof(int));
}
a[0][0] = 1;
a[0][1] = 2;
a[0][2] = 3;
a[0][3] = 4;
a[1][0] = 5;
a[1][1] = 6;
a[1][2] = 7;
a[1][3] = 8;
a[2][0] = 9;
a[2][1] = 10;
a[2][2] = 11;
a[2][3] = 12;
a[3][0] = 13;
a[3][1] = 14;
a[3][2] = 15;
a[3][3] = 16;
b[0][0] = 17;
b[0][1] = 18;
b[0][2] = 19;
b[0][3] = 20;
b[1][0] = 21;
b[1][1] = 22;
b[1][2] = 23;
b[1][3] = 24;
b[2][0] = 25;
b[2][1] = 26;
b[2][2] = 27;
b[2][3] = 28;
b[3][0] = 29;
b[3][1] = 30;
b[3][2] = 31;
b[3][3] = 32;
matrix_multiply_strassen(n, a, b, c);
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
printf("%d ", c[i][j]);
}
printf("\n");
}
for (int i = 0; i < n; i++) {
free(a[i]);
free(b[i]);
free(c[i]);
}
free(a);
free(b);
free(c);
return 0;
}
```
在此示例代码中,`matrix_add()`和`matrix_sub()`函数分别实现矩阵加法和减法,`matrix_multiply_simple()`函数实现普通的矩阵乘法,`matrix_multiply_strassen()`函数实现Strassen矩阵乘法分治法。
在`matrix_multiply_strassen()`函数中,当矩阵维度小于等于64时,使用普通矩阵乘法,否则将矩阵拆分成四个子矩阵,分别计算七个子矩阵的乘积,然后计算结果矩阵。
在`main()`函数中,我们初始化两个矩阵`a`和`b`,然后调用`matrix_multiply_strassen()`函数计算它们的乘积。最后,我们输出结果矩阵并释放内存。
注意,此示例代码仅适用于矩阵维度为2的幂次方的情况。如果矩阵维度不是2的幂次方,则需要用0填充矩阵的右下角,使其成为2的幂次方。
相关推荐
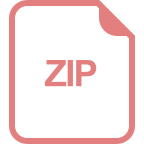













