设计新的函数,利用DES、SM4对图片进行加密和解密,能够选择加密的算法。不使用容器,使用C++openssl库,包含注释
时间: 2024-10-29 21:11:36 浏览: 28
设计一个新的函数来利用DES(Data Encryption Standard)和SM4(Stream Cipher Mode with 128-bit Key)在C++中对图片进行加密和解密,同时提供选择加密算法的功能,我们可以使用OpenSSL库。由于OpenSSL没有直接提供图像文件处理的API,我们将先读取图片数据,然后操作其二进制内容。以下是一个简单的示例:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <openssl/evp.h> // 导入OpenSSL库
// 加密函数,接受输入图片数据、算法名称("DES"或"SM4")、密钥和IV
std::vector<unsigned char> encryptImage(const std::string& imageData, const std::string& algoName, const std::string& key, const std::string& iv) {
const EVP_CIPHER* cipher = nullptr;
if (algoName == "DES") {
cipher = EVP_des_ede3_cbc();
} else if (algoName == "SM4") {
cipher = EVP_aes_128_gcm(); // SM4在OpenSSL中用AES代替,因为两者原理相似
} else {
throw std::runtime_error("Unsupported algorithm");
}
std::vector<unsigned char> encryptedData(imageData.size() + EVP_MAX_BLOCK_SIZE);
unsigned int out_len = 0;
// 创建一个临时内存上下文
BIO* bioIn = BIO_new_mem_buf((unsigned char*)imageData.c_str(), -1); // 输入流
BIO* bioOut = BIO_new(BIO_s_mem()); // 输出流
// 初始化加密上下文
EVP_CIPHER_CTX* ctx = EVP_CIPHER_CTX_new();
if (!EVP_EncryptInit_ex(ctx, cipher, NULL, reinterpret_cast<const unsigned char*>(key.c_str()), reinterpret_cast<const unsigned char*>(iv.c_str()))) {
BIO_free_all(bioIn);
BIO_free_all(bioOut);
EVP_CIPHER_CTX_free(ctx);
throw std::runtime_error("Failed to initialize encryption context");
}
// 加密数据
if (!EVP_EncryptUpdate(ctx, &encryptedData, &out_len, bioIn->data, bioIn->length)) {
BIO_free_all(bioIn);
BIO_free_all(bioOut);
EVP_CIPHER_CTX_free(ctx);
throw std::runtime_error("Encryption failed");
}
out_len += EVP_EncryptFinal_ex(ctx, &encryptedData[out_len], &out_len);
// 清理并释放资源
BIO_free_all(bioIn);
BIO_free_all(bioOut);
EVP_CIPHER_CTX_free(ctx);
encryptedData.resize(out_len);
return encryptedData;
}
// 解密函数,类似加密函数
std::vector<unsigned char> decryptImage(const std::vector<unsigned char>& encryptedData, const std::string& algoName, const std::string& key, const std::string& iv) {
// ...此处代码与encryptImage中的加密部分相反...
}
int main() {
try {
// 读取图片数据,这里假设有个本地图片文件
std::ifstream inputFile("image.jpg", std::ios::binary);
std::string imageData((std::istreambuf_iterator<char>(inputFile)), {});
// 设置加密参数
std::string algo = "DES"; // 或者 "SM4"
std::string key = "your_key";
std::string iv = "your_iv";
// 加密
auto encryptedImageData = encryptImage(imageData, algo, key, iv);
// 存储或输出加密后的数据
std::ofstream outputFile("encrypted_image.bin", std::ios::binary);
outputFile.write(reinterpret_cast<char*>(encryptedImageData.data()), encryptedImageData.size());
outputFile.close();
// 解密
auto decryptedImageData = decryptImage(encryptedImageData, algo, key, iv);
// 将解密后的数据写回图片文件
std::ofstream decryptedOutputFile("decrypted_image.jpg", std::ios::binary);
decryptedOutputFile.write(decryptedImageData.data(), decryptedImageData.size());
decryptedOutputFile.close();
std::cout << "Decryption successful!" << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
阅读全文
相关推荐
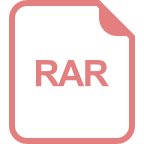
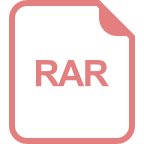
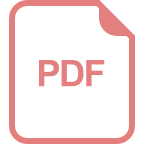
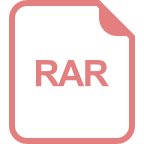
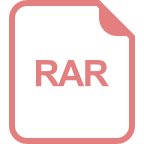
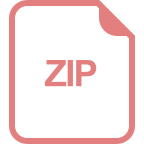
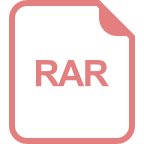
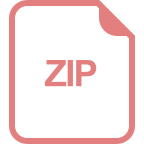
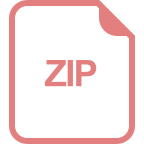
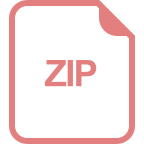
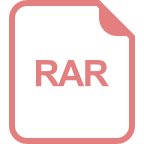
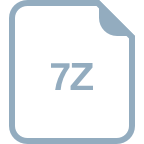
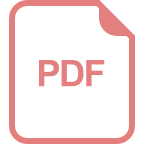
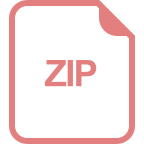
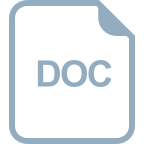
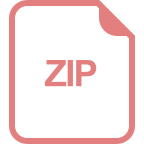
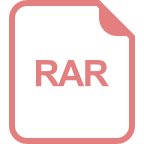