如何用C语言编程实现一个简单的图书管理系统?
时间: 2024-12-24 20:18:08 浏览: 3
在C语言中实现一个简单的图书管理系统,你可以考虑以下几个步骤:
1. 定义数据结构:首先创建一个图书结构体,包含书名(book_name)、作者(author)、出版社(publisher)和库存量(stock)等字段。
```c
typedef struct {
char book_name[100];
char author[50];
char publisher[50];
int stock;
} Book;
```
2. 功能函数:设计基本的功能,如添加新书、查询书籍信息、更新库存和删除书籍等。
```c
void add_book(Book* books, int id, const char* book_name, const char* author, const char* publisher, int stock) {
// ...添加书籍到数组或链表中...
}
Book* search_book(Book* books, int id) {
// ...遍历查找指定id的书籍...
}
void update_stock(Book* books, int id, int new_stock) {
// ...更新指定id书籍的库存...
}
void delete_book(Book* books, int id) {
// ...从数组或链表中移除指定id的书籍...
}
```
3. 用户界面:编写控制台程序,提供用户交互功能,例如菜单选项提示用户选择操作。
```c
int main() {
Book books[100]; // 假设最大有100本书
int choice;
while (1) {
printf("1. 添加新书\n");
printf("2. 查询书籍信息\n");
printf("3. 更新库存\n");
printf("4. 删除书籍\n");
printf("5. 退出\n");
scanf("%d", &choice);
switch (choice) {
case 1: // ...处理添加书籍逻辑...
break;
// 其他选项...
}
}
return 0;
}
```
阅读全文
相关推荐
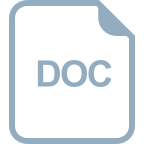
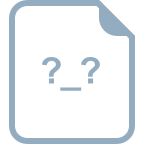
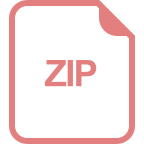















