如何用python的os库调用软件求解器
时间: 2024-04-21 22:22:55 浏览: 14
要使用Python的`os`库调用软件求解器,你可以按照以下步骤进行操作:
1. 首先,确保已经安装了需要调用的软件求解器,并且该求解器提供了命令行接口。
2. 导入`os`库。
3. 构建你需要传递给软件求解器的命令行参数。
4. 使用`os.system()`函数调用软件求解器,并将命令行参数作为字符串传递给它。
5. 获取求解器的输出结果。你可以使用Python代码来处理和分析输出结果。
下面是一个示例代码,演示了如何使用Python的`os`库调用软件求解器:
```python
import os
# 构建命令行参数
input_file = "path/to/input/file"
output_file = "path/to/output/file"
command = "solver_executable -input {} -output {}".format(input_file, output_file)
# 调用软件求解器
os.system(command)
# 处理输出结果
with open(output_file, "r") as f:
result = f.read()
# 打印输出结果
print(result)
```
请根据你使用的具体求解器和需求进行相应的调整。记得替换示例代码中的`"path/to/input/file"`、`"path/to/output/file"`和`solver_executable`为实际的文件路径和求解器可执行文件的名称。
相关问题
如何用python调用软件求解器
要用Python调用软件求解器,你可以按照以下步骤进行操作:
1. 首先,确保你已经安装了需要调用的软件求解器,并且该求解器提供了Python API或命令行接口。
2. 导入所需的Python库,比如`subprocess`或`os`库,用于执行外部命令。
3. 构建你需要传递给软件求解器的输入参数。这可以包括文件路径、命令行参数等。
4. 使用`subprocess`库中的函数(如`subprocess.run()`)或`os`库中的函数(如`os.system()`)来调用软件求解器,并将输入参数传递给它。
5. 获取求解器的输出结果。根据求解器的返回值,你可以使用Python代码来处理和分析输出结果。
下面是一个示例代码,演示了如何使用Python调用软件求解器(假设软件求解器是通过命令行接口进行调用的):
```python
import subprocess
# 构建输入参数
input_file = "path/to/input/file"
output_file = "path/to/output/file"
command = ["solver_executable", "-input", input_file, "-output", output_file]
# 调用软件求解器
subprocess.run(command)
# 处理输出结果
with open(output_file, "r") as f:
result = f.read()
# 打印输出结果
print(result)
```
请根据你使用的具体求解器和需求进行相应的调整。记得替换示例代码中的`"path/to/input/file"`、`"path/to/output/file"`和`solver_executable`为实际的文件路径和求解器可执行文件的名称。
python通过 os库调用SVN命令行
可以使用以下代码:
```python
import os
# 定义svn命令
svn_command = "svn --version"
# 调用os库执行命令
os.system(svn_command)
```
以上代码可以执行SVN命令行,并输出版本信息。如果需要执行其他SVN命令,只需要更改`svn_command`变量的值即可。
相关推荐
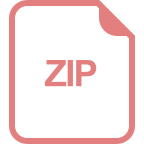












