Expected all tensors to be on the same device, but found at least two devices, cpu and cuda:
时间: 2023-11-04 19:58:25 浏览: 83
这个错误通常是由于混合使用CPU和GPU导致的。当你的代码中存在不同设备上的张量时,需要确保它们在同一设备上进行操作。你可以使用`.to(device)`方法将所有的变量和模型搬移到同一设备上。
例如,你可以使用以下代码将所有的张量搬移到GPU上进行计算:
```
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
g = g.to(device)
model = model.to(device)
```
这样,你就可以确保所有的张量都在同一设备上,避免了出现"Expected all tensors to be on the same device, but found at least two devices"的错误。
相关问题
Expected all tensors to be on the same device, but found at least two devices, cpu and cuda:0
这个错误提示通常是因为在使用PyTorch时,有些张量被放置在CPU上,有些张量被放置在GPU上,而在某些操作中需要所有的张量都在同一个设备上。为了解决这个问题,你需要将所有的张量都移动到同一个设备上。
你可以使用`.to()`方法将张量移动到指定设备,例如:
```
import torch
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
tensor1 = torch.tensor([1, 2, 3]).to(device) # 将张量tensor1移动到指定设备
tensor2 = torch.tensor([4, 5, 6]).to(device) # 将张量tensor2移动到指定设备
# 此时两个张量都在同一个设备上,不会出现Expected all tensors to be on the same device的错误
```
Expected all tensors to be on the same device, but found at least two devices, cpu and cuda:0!
这个错误通常是因为你的代码中涉及了不同设备上的张量操作。在PyTorch中,所有涉及张量的操作都要求所有张量位于同一设备上。如果你的代码中存在不同设备的张量,你可以使用`.to(device)`方法将其移动到指定设备上。
例如,你可以使用以下代码将所有张量移动到GPU上:
```python
import torch
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
# 示例张量
tensor1 = torch.tensor([1, 2, 3]).to(device)
tensor2 = torch.tensor([4, 5, 6]).to(device)
# 确保两个张量位于相同的设备上
assert tensor1.device == tensor2.device
# 进行张量操作
result = tensor1 + tensor2
print(result)
```
在这个例子中,如果你的计算机上有可用的GPU,则张量将被移动到GPU上进行操作。否则,它们将保留在CPU上进行操作。
阅读全文
相关推荐
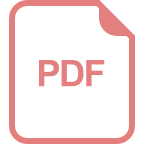
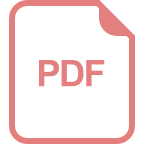
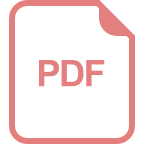









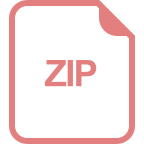
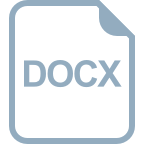
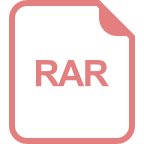
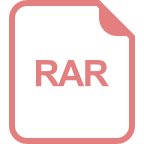
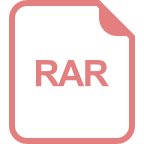