在Unity里面如何实现人物靠近宝箱,宝箱自动打开的动画效果播放,且宝箱只能打开一次,在代码里面怎么写?用简单易懂的代码语言来实现,且在Unity里面的操作也该如何
时间: 2024-12-20 16:18:11 浏览: 9
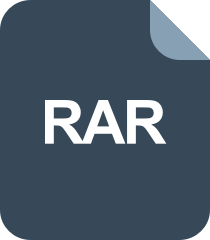
UiFrameAnimator_unity播放序列帧源码_
在Unity中实现这样的动画效果,首先需要创建两个脚本:一个是角色(Character)的,另一个是宝箱( Chest)的。这里我会用C#编写简单的示例:
1. **角色脚本 (CharacterController)**:
```csharp
public class Character : MonoBehaviour
{
public GameObject chest; // 宝箱游戏物体引用
private bool chestOpened = false;
void OnTriggerEnter(Collider other)
{
if (!chestOpened && other.gameObject.CompareTag("Chest"))
{
StartCoroutine(AnimateToOpen());
chestOpened = true;
}
}
IEnumerator AnimateToOpen()
{
while (true) // 播放打开动画
{
chest.GetComponent<Animator>().SetTrigger("Open");
yield return new WaitForSeconds(0.5f); // 动画持续时间,比如0.5秒
if (chest.GetComponent<Animator>().IsFinished) break; // 如果动画完成就跳出循环
}
}
}
```
这个脚本会检查碰撞事件,当角色接近并触碰到“Chest”标签的宝箱时,开始播放宝箱的打开动画。
2. **宝箱脚本 (Chest)**:
```csharp
public class Chest : MonoBehaviour, IAnimatorListener
{
private Animator animator;
void Start()
{
animator = GetComponent<Animator>();
animator.AddListener(this);
}
public void OnAnimatorIK(int layerIndex, AnimationEvent animationEvent)
{
if (animationEvent.name == "Open" && animationEvent.phase == AnimatorPhase.Finished)
{
// 当动画结束,关闭宝箱无法再次触发
animator.SetTrigger("Closed");
DisableInteractions();
}
}
void DisableInteractions() // 防止多次触发打开动画
{
// 这里可以设置宝箱交互组件不可用或者禁用碰撞检测等
}
}
```
这个脚本监听自身动画是否完成,当动画结束后,触发关闭动画并禁止进一步的互动。
阅读全文
相关推荐
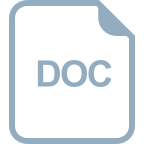
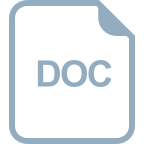

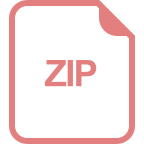
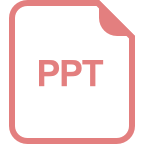
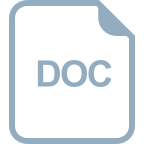
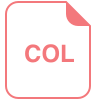
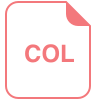
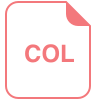








