编写python程序,输入n个分数,输出最高分,最低分和平均分
时间: 2024-10-25 08:14:47 浏览: 9
在Python中,你可以通过列表来存储用户输入的分数,然后遍历这个列表找到最高分、最低分,并计算平均值。以下是一个简单的示例:
```python
def get_scores():
n = int(input("请输入分数的数量:"))
scores = [] # 创建一个空列表来存放分数
for i in range(n):
score = float(input(f"请输入第{i+1}个分数:"))
scores.append(score) # 将每个分数添加到列表中
highest_score = max(scores)
lowest_score = min(scores)
average_score = sum(scores) / n # 计算平均值
print(f"最高分是:{highest_score}")
print(f"最低分是:{lowest_score}")
print(f"平均分是:{average_score}")
get_scores()
```
在这个程序中,我们首先让用户输入分数的数量`n`,然后循环`n`次,每次获取一个分数并加入到列表中。最后,我们使用内置函数`max()`和`min()`找出最高分和最低分,通过列表推导式计算平均值。
相关问题
python 编写程序,输入n个分数(n<=100),输出最高分、最低分和平均分
下面是一个简单的 Python 程序,可以实现输入 n 个分数,然后输出最高分、最低分和平均分:
```python
n = int(input("请输入分数的个数:"))
scores = []
for i in range(n):
score = float(input("请输入第%d个分数:" % (i+1)))
scores.append(score)
max_score = max(scores)
min_score = min(scores)
avg_score = sum(scores) / n
print("最高分:%.2f" % max_score)
print("最低分:%.2f" % min_score)
print("平均分:%.2f" % avg_score)
```
程序首先让用户输入分数的个数,然后使用一个循环输入具体的分数,将分数存储在一个列表 `scores` 中。使用 Python 内置的 `max()`、`min()` 和 `sum()` 函数可以很方便地计算出最高分、最低分和平均分。最后,使用 `print()` 函数输出结果。注意,使用 `%.2f` 可以控制输出的小数位数为两位。
python 编写程序,输入n个分数(n<=100),输出最高分、最低分和平均分。
可以使用以下代码实现:
```python
n = int(input("请输入分数的个数:"))
scores = []
for i in range(n):
score = float(input("请输入第{}个分数:".format(i+1)))
scores.append(score)
max_score = max(scores)
min_score = min(scores)
avg_score = sum(scores) / n
print("最高分为:{:.2f}".format(max_score))
print("最低分为:{:.2f}".format(min_score))
print("平均分为:{:.2f}".format(avg_score))
```
具体思路是,首先输入分数的个数n,然后循环输入n个分数,将它们存储在一个列表scores中。接着使用max函数和min函数分别求出最高分和最低分,使用sum函数求出分数总和,再除以n求出平均分。最后使用格式化字符串输出结果。
阅读全文
相关推荐
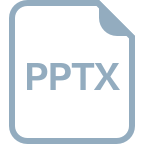













