Android Studio如何处理网络请求以获取植物信息?
时间: 2024-12-11 07:38:19 浏览: 9
在Android Studio中,处理网络请求通常会使用诸如Retrofit、OkHttp、Volley或Kotlin Coroutines这样的库。以下是使用Retrofit作为例子的一个简要步骤:
1. 添加依赖:首先,在build.gradle文件中添加Retrofit和Gson或Moshi(取决于你想使用的数据解析库)的依赖。
```gradle
dependencies {
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.squareup.retrofit2:converter-gson:2.9.0' // 或者 'com.squareup.moshi:moshi-kotlin:1.14.0'
}
```
2. 创建接口:定义一个代表植物信息API的接口,例如`PlantApiService.kt`。
```kotlin
interface PlantApiService {
@GET("plants")
suspend fun getPlantInfo(): List<Plant>
}
```
3. 客户端配置:创建一个Retrofit实例,并指定基础URL。
```kotlin
val retrofit = Retrofit.Builder()
.baseUrl("https://api.example.com/")
.addConverterFactory(GsonConverterFactory.create()) // 或者 MoshiConverterFactory.create()
.build()
val plantApiService = retrofit.create(PlantApiService::class.java)
```
4. 发送请求并处理结果:在需要获取植物信息的地方,使用Kotlin的suspend函数和Coroutine来发起异步请求。
```kotlin
fun fetchPlantInfo() = withContext(Dispatchers.IO) {
try {
val plants = plantApiService.getPlantInfo()
// 对响应的数据进行处理,如显示在UI上
plants.forEach { plant ->
// do something with plant data
}
} catch (e: Exception) {
logError("Failed to fetch plants", e)
}
}
```
阅读全文
相关推荐
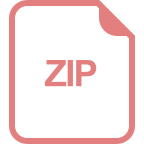
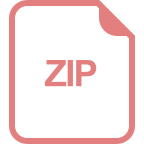

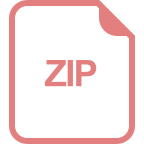
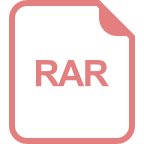
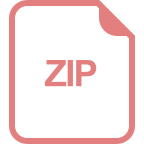
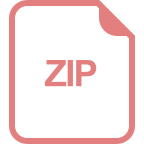
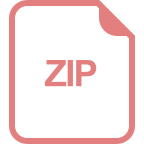
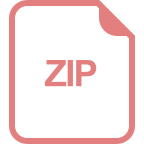
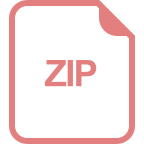
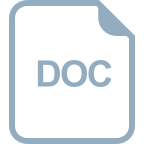
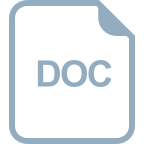
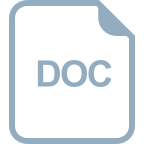
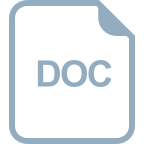
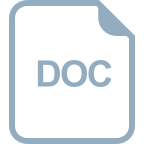
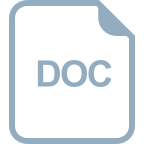
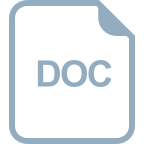

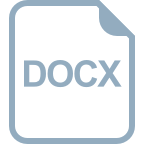