如何在Android Studio中使用适当的库和方法来处理网络请求,以便从互联网上获取植物相关的详细信息?
时间: 2024-12-11 16:37:01 浏览: 9
在Android Studio中,处理网络请求通常会涉及到使用HTTP客户端库,如OkHttp、Retrofit或者Volley等。以下是使用Retrofit(一个高层次的网络请求库,基于OkHttp)的一个简单示例:
1. **添加依赖**:
- 首先,你需要在`build.gradle(Module)`文件中添加Retrofit和Gson(用于序列化JSON数据)的依赖:
```groovy
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.squareup.retrofit2:converter-gson:2.9.0'
```
2. **创建接口**:
创建一个代表API服务的接口,例如获取植物信息的接口:
```java
public interface PlantApi {
@GET("plants")
Call<List<Plant>> getPlants(@Query("id") String plantId);
}
```
3. **创建Repository**:
实现这个接口,并设置Retrofit实例:
```java
class PlantRepository {
private final PlantApi plantApi;
public PlantRepository(PlantApi plantApi) {
this.plantApi = plantApi;
}
public LiveData<List<Plant>> fetchPlantInfo(String plantId) {
return new MutableLiveData<>(fetchFromNetwork(plantId));
}
private List<Plant> fetchFromNetwork(String plantId) {
Call<List<Plant>> call = plantApi.getPlants(plantId);
call.enqueue(new Callback<List<Plant>>() {
@Override
public void onResponse(Call<List<Plant>> call, Response<List<Plant>> response) {
if (response.isSuccessful()) {
plantList.postValue(response.body());
} else {
// 处理错误
}
}
@Override
public void onFailure(Call<List<Plant>> call, Throwable t) {
// 处理网络异常
}
});
return plantList;
}
}
```
4. **在ViewModel中使用**:
在ViewModel中注入Repository并发起网络请求:
```java
class PlantDetailsViewModel extends ViewModel {
private final PlantRepository repository;
public PlantDetailsViewModel(PlantRepository repository) {
this.repository = repository;
}
public void getPlantDetails(String plantId) {
repository.fetchPlantInfo(plantId).observe(this, new Observer<List<Plant>>() {
@Override
public void onChanged(List<Plant> plants) {
// 处理获取到的数据
}
});
}
}
```
阅读全文
相关推荐
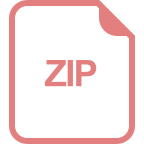
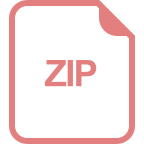

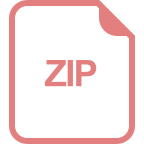
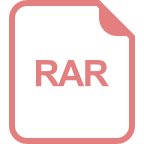
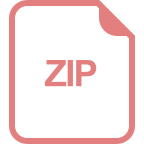
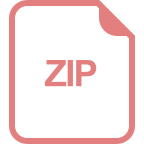
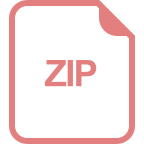
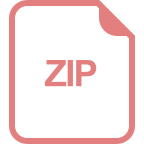
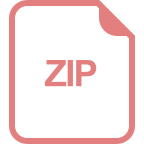
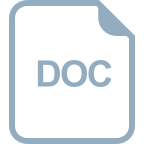
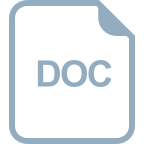
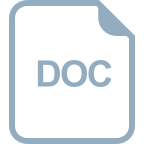
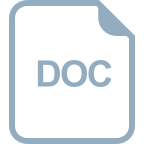
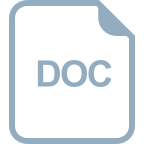
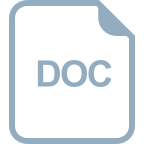
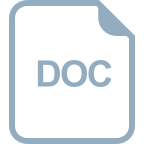
