Android开发 考试系统记录当前题号
时间: 2024-09-12 16:14:15 浏览: 32
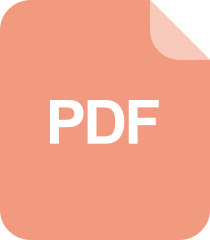
用Authorware软件开发考试系统
在Android开发中,如果要设计一个考试系统来记录当前的题号,通常会涉及到数据存储和UI更新两部分。你可以采用以下几个步骤:
1. 数据模型:创建一个`Question`类,包含字段如`id`、`questionText`和`isCurrent`等,其中`isCurrent`标记是否当前正在答题。
```java
public class Question {
private int id;
private String questionText;
private boolean isCurrent;
// getters and setters...
}
```
2. 数据存储:使用SQLite数据库或者其他合适的数据存储方案来保存题目信息,每次切换到新题时将上一题设置为非current,同时更新当前题目的isCurrent属性。
```java
DatabaseHelper dbHelper = new DatabaseHelper(context);
Question currentQuestion = dbHelper.getCurrentQuestion();
// 切换到新题
Question nextQuestion = getNextQuestion();
dbHelper.updateQuestion(currentQuestion.setId(nextQuestion.getId()), isCurrent: false);
dbHelper.insertOrUpdateQuestion(nextQuestion, isCurrent: true);
```
3. UI更新:在展示题目列表的地方,通过查询数据库获取所有题目,并在相应的item上显示当前题号。当用户选择下一题时,更新UI并调用记录当前题号的相关操作。
```java
RecyclerView recyclerView = findViewById(R.id.recycler_view_questions);
recyclerView.setAdapter(new ExamAdapter(questionList));
ExamAdapter.OnItemClickListener onItemClick = (position) -> {
if (position < questionList.size()) {
Question selectedQuestion = questionList.get(position);
updateInterface(selectedQuestion);
}
};
```
4. 更新界面:在`updateInterface(Question question)`方法中,你可以设置题目视图的文本,并更新UI组件的状态。
```java
private void updateInterface(Question question) {
textViewQuestion.setText(question.questionText);
// 如果需要,还可以更改其他视图的样式或颜色,如高亮已答题目
if (question.isCurrent) {
textViewQuestion.setBackgroundColor(ContextCompat.getColor(context, R.color.current_question_color));
} else {
textViewQuestion.setBackgroundResource(0); // 清除背景色
}
}
```
阅读全文
相关推荐
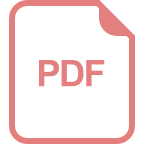
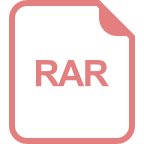
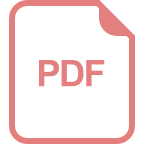
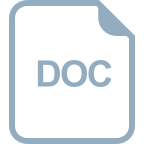
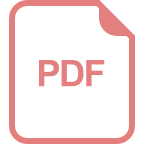
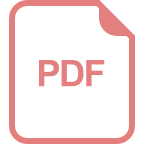
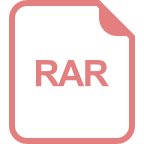
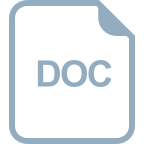
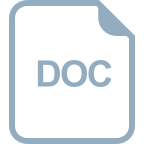
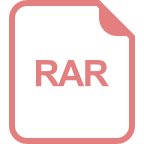
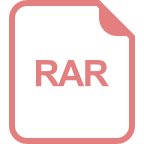
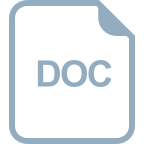


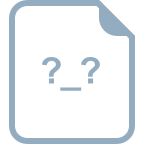