c++实现1. 文件读取功能: 从文件中读取关键字。 如: We could not go for a walk that afternoon. There was such a freezing cold winter, … 读取文件时只通过空格分隔,即有空格分隔即为一个单词。不区分大小写,即We与we认为是同一关键词。为了减少预处理操作,可将文件中逗号、句号等提前去除。 2. 存储功能: 设计存储结构,将从文件中读取的数据存储到计算机中。 3. 查找功能: 根据关键词进行查找,记录预处理时间和查找时间。预处理时间包括从文件中读取并存储的时间。 时间(秒) 100 1000 1万 10万 100万 顺序查找法 二分查找法 散列表法 二叉检索树法 平衡树法 …
时间: 2024-04-14 15:27:33 浏览: 21
这是一个实现文件读取、存储和查找关键字的功能的C++程序。首先,我们需要定义一个数据结构来存储关键字,并实现文件读取功能。然后,我们可以使用不同的查找算法来查找关键字,并记录预处理时间和查找时间。
以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <unordered_set>
#include <chrono>
using namespace std;
struct KeywordStorage {
unordered_set<string> keywords;
void readFromFile(const string& filename) {
ifstream file(filename);
if (!file.is_open()) {
cout << "Failed to open file: " << filename << endl;
return;
}
string line;
while (getline(file, line)) {
// 去除逗号、句号等标点符号
line.erase(remove_if(line.begin(), line.end(), ::ispunct), line.end());
istringstream iss(line);
string word;
while (iss >> word) {
// 转换为小写字母
transform(word.begin(), word.end(), word.begin(), ::tolower);
keywords.insert(word);
}
}
file.close();
}
};
int main() {
KeywordStorage storage;
storage.readFromFile("input.txt");
// 在此处实现不同的查找算法,并记录预处理时间和查找时间
return 0;
}
```
在这个示例中,我们使用了unordered_set来存储关键字,它提供了O(1)的平均查找时间。你可以根据需要选择其他的数据结构和查找算法。
请注意,这只是一个简单的示例代码,你还需要根据实际需求进行适当的修改和完善。希望对你有所帮助!
相关推荐
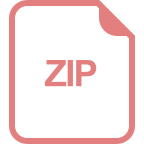















