链表的基本操作代码c++要求有操作提示
时间: 2024-09-14 15:04:25 浏览: 35
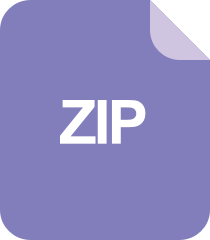
C++基于QT的链表操作演示系统源码+实验报告+详细代码注释(课设项目).zip
链表是一种常见的数据结构,它由一系列节点组成,每个节点包含数据和指向下一个节点的指针。在C++中,链表的基本操作通常包括创建链表、插入节点、删除节点和遍历链表等。
下面是一些链表基本操作的C++代码示例,每个函数都有操作提示:
```cpp
#include <iostream>
// 定义链表节点结构体
struct ListNode {
int data; // 节点数据
ListNode* next; // 指向下一个节点的指针
// 构造函数初始化节点数据和指针
ListNode(int x) : data(x), next(nullptr) {}
};
// 创建链表,这里以创建一个简单的单向链表为例
ListNode* createList(int arr[], int n) {
if(n == 0) return nullptr;
// 创建头节点
ListNode* head = new ListNode(arr[0]);
ListNode* current = head;
// 遍历数组,为每个元素创建新节点并链接
for(int i = 1; i < n; ++i) {
current->next = new ListNode(arr[i]);
current = current->next;
}
return head; // 返回链表头指针
}
// 插入节点,这里以在链表头部插入节点为例
void insertAtHead(ListNode*& head, int value) {
ListNode* newNode = new ListNode(value);
newNode->next = head;
head = newNode;
}
// 删除节点,这里以删除链表中值为value的节点为例
void deleteNode(ListNode*& head, int value) {
ListNode* current = head;
ListNode* prev = nullptr;
while(current != nullptr && current->data != value) {
prev = current;
current = current->next;
}
if(current == nullptr) return; // 没有找到值为value的节点
if(prev == nullptr) {
// 要删除的是头节点
head = current->next;
} else {
// 要删除的不是头节点
prev->next = current->next;
}
delete current; // 释放被删除节点的内存
}
// 遍历链表并打印节点数据
void printList(ListNode* head) {
ListNode* current = head;
while(current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
// 释放链表内存
void freeList(ListNode*& head) {
ListNode* temp;
while(head != nullptr) {
temp = head;
head = head->next;
delete temp;
}
}
int main() {
// 创建一个链表
int arr[] = {1, 2, 3, 4, 5};
ListNode* myList = createList(arr, 5);
std::cout << "链表元素为:";
printList(myList); // 打印链表
// 在链表头部插入新节点
insertAtHead(myList, 0);
std::cout << "插入元素0后的链表为:";
printList(myList); // 打印链表
// 删除链表中的元素
deleteNode(myList, 3);
std::cout << "删除元素3后的链表为:";
printList(myList); // 打印链表
// 释放链表内存
freeList(myList);
return 0;
}
```
阅读全文
相关推荐
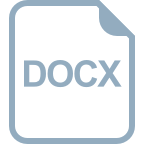
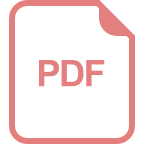
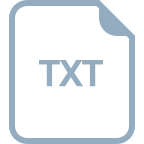
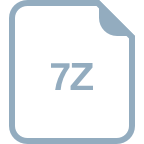
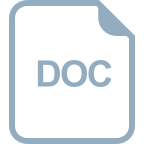
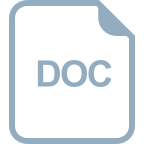
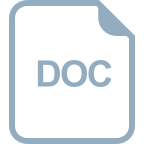
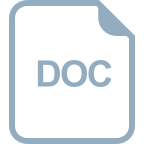
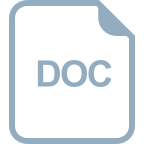
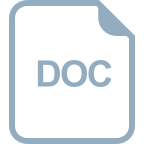
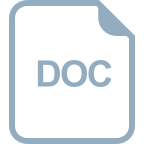
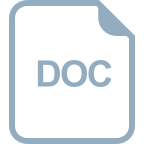
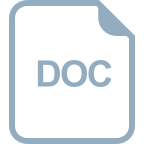
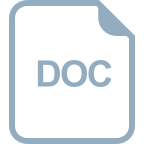
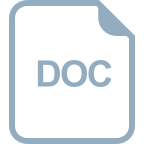
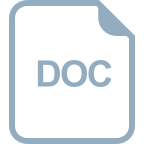
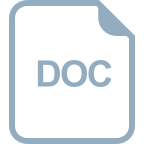

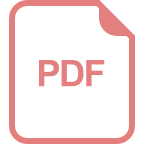