用C语言实现单链表的基本示例,包含了创建链表、求链表长度、输出链表、插入、删除和查找等功能,并且测试以上功能。
时间: 2024-09-26 07:09:45 浏览: 39
在C语言中,我们可以使用结构体和指针来实现单链表。以下是基本的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点并初始化
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 初始化空链表
Node* initList() {
Node* head = NULL;
return head;
}
// 插入元素到链表末尾
void insertAtEnd(Node** head, int value) {
Node* newNode = createNode(value);
if (*head == NULL) {
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 计算链表长度
int length(Node* head) {
int count = 0;
Node* temp = head;
while (temp != NULL) {
count++;
temp = temp->next;
}
return count;
}
// 输出链表
void printList(Node* head) {
Node* temp = head;
printf("链表内容: ");
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
// 查找元素
Node* search(Node* head, int value) {
Node* current = head;
while (current != NULL) {
if (current->data == value)
return current;
current = current->next;
}
return NULL;
}
// 删除节点 (仅演示了简单情况,实际删除需要考虑边界条件)
void deleteNode(Node** head, int value) {
Node* current = *head;
Node* previous = NULL;
if (current != NULL && current->data == value) {
*head = current->next;
free(current);
return;
}
while (current != NULL && current->data != value) {
previous = current;
current = current->next;
}
if (current != NULL) {
previous->next = current->next;
free(current);
}
}
int main() {
Node* list = initList();
// 测试功能
insertAtEnd(&list, 1);
insertAtEnd(&list, 2);
insertAtEnd(&list, 3);
printList(list); // 应该输出: 链表内容: 1 2 3
printf("链表长度: %d\n", length(list)); // 应该输出: 链表长度: 3
Node* found = search(list, 2);
if (found != NULL)
printf("找到了值2的节点: %d\n", found->data);
else
printf("未找到值2的节点。\n");
deleteNode(&list, 2);
printList(list); // 删除后应输出: 链表内容: 1 3
return 0;
}
```
这个程序展示了如何创建、操作和测试一个简单的单链表。请注意,在实际项目中,删除节点通常会更复杂一些,因为它涉及到处理前驱节点的情况。
阅读全文
相关推荐
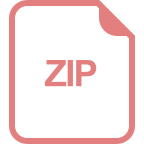
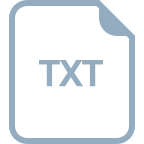
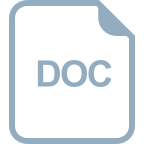




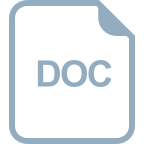
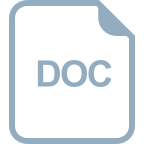
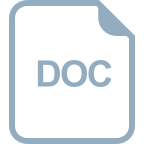
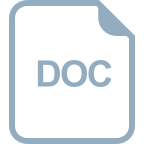
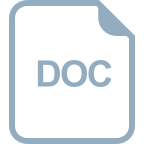
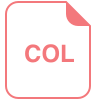
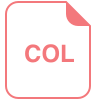
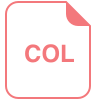
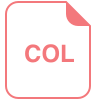


