linux c++ 删除文件
时间: 2023-11-21 10:50:41 浏览: 240
在 Linux C++ 中,可以使用标准库中的 remove 函数来删除文件。具体用法如下:
```c++
#include <cstdio>
int main() {
const char* filename = "test.txt";
if (std::remove(filename) != 0) {
std::perror("Error deleting file");
} else {
std::puts("File successfully deleted");
}
return 0;
}
```
在上面的代码中,我们首先定义了要删除的文件名为 "test.txt",然后调用 remove 函数来删除该文件。如果 remove 函数返回值不为 0,则说明删除失败,我们可以使用 perror 函数来输出错误信息;否则,说明删除成功,我们可以使用 puts 函数来输出成功信息。
相关问题
linux c++ 删除文件内容
可以使用 C++ 的 fstream 库来删除文件内容。具体步骤如下:
1. 打开文件,以只写模式打开(ios::out)。
2. 将文件指针移动到文件末尾(ios::ate)。
3. 将文件长度截为0(truncate)。
4. 关闭文件。
以下是示例代码:
```c++
#include <fstream>
#include <iostream>
int main() {
std::ofstream ofs("example.txt", std::ios::out | std::ios::ate);
ofs.seekp(0, std::ios::beg);
ofs << "";
ofs.close();
return 0;
}
```
linux c++ 如何删除文件?
在Linux下,C++中删除文件通常使用`std::remove()`函数配合`std::error_code`来处理可能存在的权限问题。如果遇到不可删除的文件(比如具有只读权限),你可能需要先改变其权限或者提升当前用户的权限。以下是一个基本示例:
```cpp
#include <iostream>
#include <filesystem>
// 使用filesystem库删除文件
int main() {
try {
std::filesystem::path file_to_remove("/path/to/your/file");
if (!std::filesystem::exists(file_to_remove)) {
std::cerr << "File does not exist." << std::endl;
} else {
std::error_code ec;
if (std::filesystem::remove(file_to_remove, ec)) {
if (ec.value()) {
std::cerr << "Failed to remove file: " << ec.message() << std::endl;
} else {
std::cout << "File removed successfully." << std::endl;
}
} else {
std::cout << "File already deleted or could be removed without error." << std::endl;
}
}
} catch (const std::exception& e) {
std::cerr << "Error occurred: " << e.what() << std::endl;
}
return 0;
}
```
注意,这段代码假设你有权限访问并删除指定的文件。如果权限不足,`std::filesystem::remove()`可能会返回错误代码,这时你可以根据具体情况进行相应的处理。
阅读全文
相关推荐
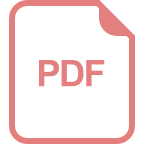
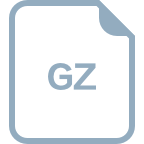
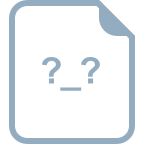
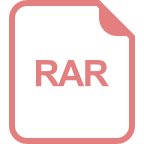
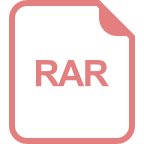
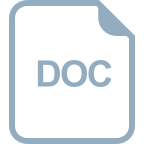
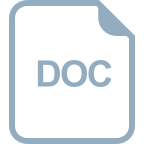




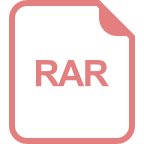
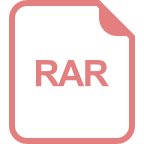
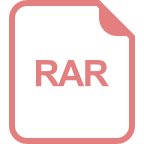
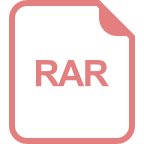