\t和\r作用(代码举例说明)
时间: 2024-04-17 13:21:10 浏览: 9
\t和\r是两个特殊的转义字符,它们在编程中常用于控制字符的显示和换行操作。
1. \t:表示水平制表符,用于在输出中创建水平间距。在字符串中使用\t可以将文本对齐到下一个制表符位置。例如,以下是一个使用\t的代码示例:
```python
print("姓名\t年龄\t城市")
print("张三\t20\t北京")
print("李四\t25\t上海")
```
输出结果:
```
姓名 年龄 城市
张三 20 北京
李四 25 上海
```
2. \r:表示回车符,用于将光标移动到当前行的开头位置。在字符串中使用\r可以实现文本的覆盖输出。例如,以下是一个使用\r的代码示例:
```python
import time
for i in range(5):
print("倒计时:", 5-i, end="\r")
time.sleep(1)
print("倒计时结束!")
```
输出结果:
```
倒计时结束!
```
相关问题
请使用代码示例,举例说明圆弧插补的作用
圆弧插补可以实现机床在加工曲线轮廓时的精确控制,以下是一个简单的圆弧插补的代码示例:
```c++
#include <iostream>
#include <cmath>
using namespace std;
int main()
{
double x0 = 0, y0 = 0; // 起点坐标
double x1 = 2, y1 = 2; // 终点坐标
double cx = 1, cy = 0; // 圆心坐标
double r = sqrt((x1-cx)*(x1-cx) + (y1-cy)*(y1-cy)); // 半径
// 从起点到圆弧起点的直线插补
for (double t = 0; t < 1; t += 0.1) {
double x = x0 + t * (cx - x0);
double y = y0 + t * (cy - y0);
cout << "Move to (" << x << ", " << y << ")" << endl;
}
// 圆弧插补
for (double t = 0; t < 1; t += 0.1) {
double angle = t * M_PI / 2;
double x = cx + r * cos(angle);
double y = cy + r * sin(angle);
cout << "Line to (" << x << ", " << y << ")" << endl;
}
// 从圆弧终点到终点的直线插补
for (double t = 0; t < 1; t += 0.1) {
double x = cx + t * (x1 - cx);
double y = cy + t * (y1 - cy);
cout << "Line to (" << x << ", " << y << ")" << endl;
}
return 0;
}
```
这个代码示例实现了从起点到终点的圆弧插补,先沿着起点到圆弧起点的直线插补一段距离,然后在圆弧上插补一段圆弧路径,最后再沿着圆弧终点到终点的直线插补一段距离。这样就可以精确地控制机床在加工曲线轮廓时的路径,达到更高的加工精度。
C语言如何根据配置文件生成对应的结构体?请用代码举例说明
C语言中可以使用配置文件来存储程序的配置信息,常见的配置文件格式有INI、JSON、XML等。在程序运行时,需要将配置文件中的信息读取到程序中,并转化为对应的数据结构,方便程序进行处理。下面是一个生成结构体的示例代码,以INI文件格式为例:
假设有一个名为config.ini的配置文件,内容如下:
```
[database]
host=127.0.0.1
port=3306
username=root
password=123456
```
需要生成一个对应的结构体,可以定义如下:
```c
typedef struct {
char host[32];
int port;
char username[32];
char password[32];
} DatabaseConfig;
```
然后可以编写一个函数来读取配置文件并生成对应的结构体:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 1024
void parse_config_file(const char *filename, DatabaseConfig *config) {
FILE *fp;
char line[MAX_LINE_LEN];
char *key, *value;
if ((fp = fopen(filename, "r")) == NULL) {
fprintf(stderr, "Can't open %s\n", filename);
exit(EXIT_FAILURE);
}
while (fgets(line, MAX_LINE_LEN, fp) != NULL) {
if (line[0] == '#' || line[0] == '\n') {
continue; // 忽略注释和空行
}
key = strtok(line, "=");
value = strtok(NULL, "=");
value[strcspn(value, "\r\n")] = '\0'; // 去掉换行符
if (strcmp(key, "host") == 0) {
strcpy(config->host, value);
} else if (strcmp(key, "port") == 0) {
config->port = atoi(value);
} else if (strcmp(key, "username") == 0) {
strcpy(config->username, value);
} else if (strcmp(key, "password") == 0) {
strcpy(config->password, value);
}
}
fclose(fp);
}
```
使用示例:
```c
int main() {
DatabaseConfig config;
parse_config_file("config.ini", &config);
printf("host=%s, port=%d, username=%s, password=%s\n", config.host, config.port, config.username, config.password);
return 0;
}
```
输出结果:
```
host=127.0.0.1, port=3306, username=root, password=123456
```
相关推荐
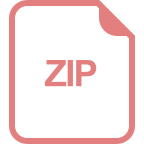
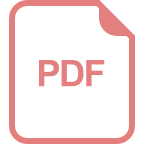








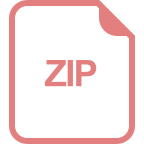
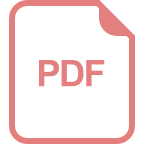
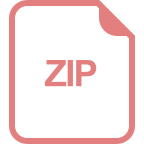
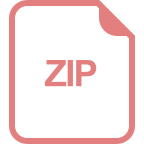
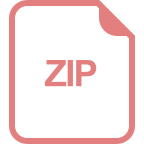
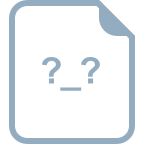