Python用序列存储学生信息并定义并调用函数实现学生信息的删除与修改
时间: 2024-10-29 15:13:11 浏览: 25
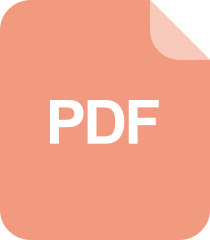
基于python实现学生信息管理系统
在Python中,你可以使用列表(list)或字典(dict)作为数据结构来存储学生的个人信息,因为它们都支持动态添加、删除和修改元素。这里我们先假设学生信息包含姓名(name)和成绩(score)。
```python
# 定义一个学生类,表示单个学生的信息
class Student:
def __init__(self, name, score):
self.name = name
self.score = score
# 使用列表存储学生信息,每个元素是一个Student实例
students = [
Student("张三", 90),
Student("李四", 85),
Student("王五", 95)
]
# 定义删除学生的方法,通过索引来访问列表中的元素
def delete_student(index):
if index < len(students):
students.pop(index)
# 定义修改学生的方法,同样需要索引来找到对应的Student对象
def update_student(index, new_name=None, new_score=None):
if index < len(students):
if new_name:
students[index].name = new_name
if new_score is not None:
students[index].score = new_score
# 调用函数示例
delete_student(1) # 删除第二个学生
update_student(0, "赵六", 92) # 修改第一个学生的信息
# 打印更新后的学生列表
for student in students:
print(f"学生:{student.name}, 成绩:{student.score}")
```
阅读全文
相关推荐
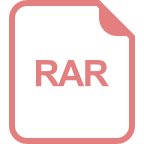
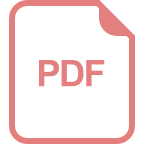
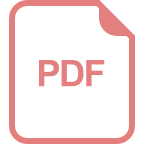
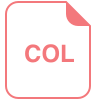


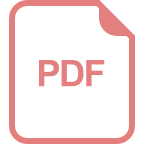
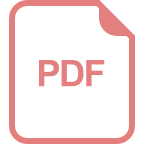
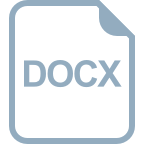
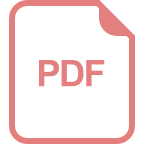
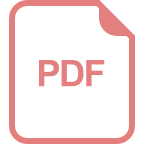
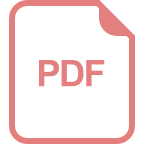
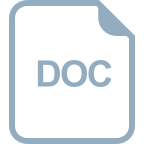
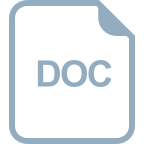



