离散的粒子群优化算法代码
时间: 2024-12-19 14:13:27 浏览: 4
离散粒子群优化(DPSO)是一种模拟鸟群觅食行为的优化算法,它将个体视为粒子,在搜索空间中寻找最优解。基本步骤包括:
1. 初始化粒子群体:创建一组随机位置和速度的粒子,每个粒子代表一个可能的解决方案。
2. 遗传编码:用粒子的位置表示解的质量,通常通过坐标值或实数范围表示。
3. 移动策略:对于每一代,每个粒子更新其速度和位置:
- **局部最优**:粒子尝试在其当前最佳位置附近找到更好的解。
- **全局最优**:粒子查看整个群体的最佳位置,并尝试向那个方向移动。
4. 更新规则:根据个人最好位置(pBest)和群体最好位置(gBest),计算新的速度和位置。
- **速度更新**:新速度 = w * 当前速度 + c1 * rand(pBest) + c2 * rand(gBest)
- **位置更新**:新位置 = 当前位置 + 新速度
5. 判断收敛:检查是否达到预设的迭代次数或解的质量满足某个阈值,若未达则继续循环;否则停止并返回gBest作为结果。
编写DPSO算法的具体代码取决于你使用的编程语言,例如Python可以这样简化:
```python
import numpy as np
def dpso(pop_size, max_iter, bounds, w, c1, c2):
particles = np.random.uniform(bounds[:, 0], bounds[:, 1], (pop_size, len(bounds)))
p_best = particles.copy()
g_best = particles[np.argmin(particles.sum(axis=1))]
for _ in range(max_iter):
r1, r2 = np.random.rand(pop_size, 1), np.random.rand(pop_size, 1)
velocities = w * velocities + c1 * r1 * (p_best - particles) + c2 * r2 * (g_best - particles)
positions = velocities + particles
# 检查新位置是否超出边界,如果超出,则还原到边界内
positions = np.clip(positions, bounds[:, 0], bounds[:, 1])
# 更新局部和个人最优
fitnesses = evaluate_fitness(positions) # 假设evaluate_fitness是一个函数评估每个解的适应度
best_indices = np.argsort(fitnesses)
particles[best_indices] = positions[best_indices]
p_best[best_indices] = positions[best_indices]
if fitnesses[0] < g_best[0]:
g_best = positions[0]
return g_best
# 函数假设你需要根据粒子的位置计算适应度
def evaluate_fitness(positions):
# ... (根据具体问题计算适应度)
```
阅读全文
相关推荐
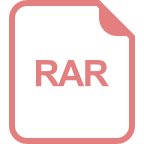
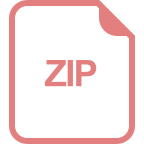
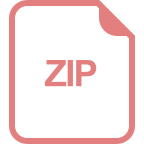
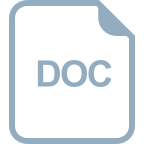
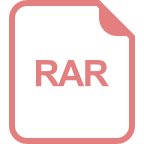
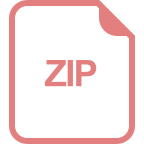
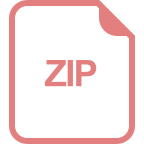
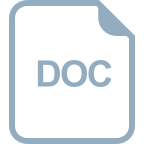
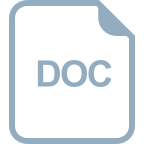
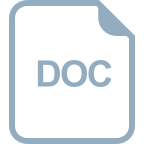

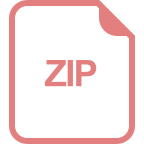
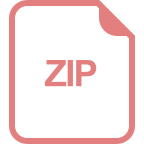
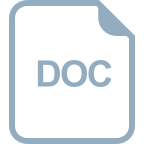
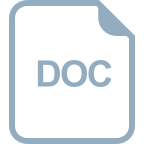
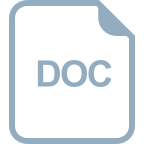
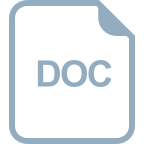
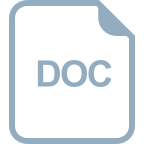