yolov5调用摄像头代码
时间: 2023-11-20 20:54:02 浏览: 136
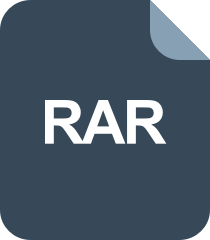
yolov5调用摄像头

以下是使用yolov5调用摄像头的代码:
```python
python detect.py --weights runs/train/exp/weights/best.pt --save-txt --source='2'
```
其中,--weights参数指定了训练好的权重文件路径,--save-txt参数表示保存检测结果到txt文件中,--source参数指定了摄像头的序号,这里是外接摄像头的序号。
需要注意的是,这个序号是一个字符串,且代表的就是外接摄像头的序号。
阅读全文
相关推荐

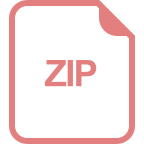











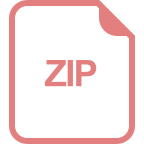
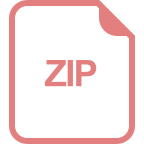