设计一个算法用VS2015编译器C语言版完成单链表的建立、取值、查找、插入和删除操作。单链表存储元素为int的数据元素,取值范围1到900。
时间: 2024-10-08 14:11:26 浏览: 30
为了设计一个简单的单链表数据结构并实现相应的操作,我们可以创建以下几个关键类:
1. **节点** (Node):用于表示链表中的每个元素,包含整数值和指向下一个节点的指针。
```c
typedef struct Node {
int data; // 存储的整数
struct Node* next; // 指向下一个节点的指针
} Node;
```
2. **链表** (LinkedList):包含头节点和辅助函数。
```c
typedef struct LinkedList {
Node* head; // 链表头部节点
} LinkedList;
// 初始化链表为空
void createEmptyList(LinkedList* list) {
list->head = NULL;
}
// 插入新元素
void insert(int value, LinkedList* list) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = list->head;
list->head = newNode;
}
// 取值
int getValue(const LinkedList* list, int index) {
if (index < 0 || !list->head || index >= getLength(list)) {
return -1; // 表示越界或无效索引
}
Node* curr = list->head;
for (int i = 0; i < index; ++i) {
curr = curr->next;
}
return curr->data;
}
// 查找元素
Node* findElement(int value, const LinkedList* list) {
Node* curr = list->head;
while (curr != NULL) {
if (curr->data == value) {
return curr;
}
curr = curr->next;
}
return NULL; // 如果找不到,则返回NULL
}
// 删除元素
void deleteElement(int value, LinkedList* list) {
if (!list->head) return;
if (list->head->data == value) {
Node* temp = list->head;
list->head = list->head->next;
free(temp);
return;
}
Node* prev = list->head;
Node* curr = list->head->next;
while (curr != NULL) {
if (curr->data == value) {
prev->next = curr->next;
free(curr);
return;
}
prev = curr;
curr = curr->next;
}
}
// 获取链表长度
int getLength(const LinkedList* list) {
int length = 0;
Node* curr = list->head;
while (curr != NULL) {
++length;
curr = curr->next;
}
return length;
}
```
阅读全文
相关推荐
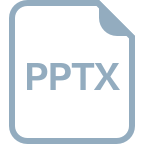
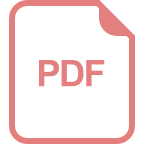
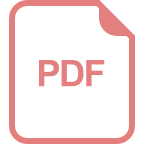

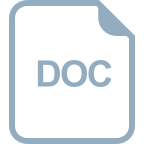
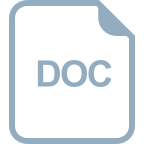
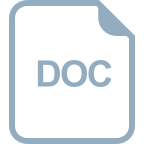
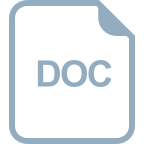
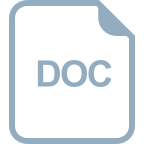
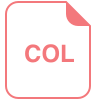
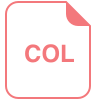
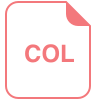
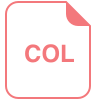
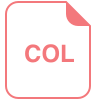
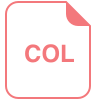
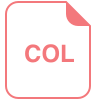
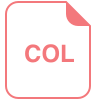
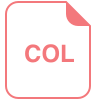
