html在线考试系统
时间: 2023-11-24 14:53:03 浏览: 74
以下是一个使用纯HTML、JS和CSS编写的在线考试系统的示例:
```html
<!DOCTYPE html>
<html>
<head>
<title>在线考试系统</title>
<style>
body {
font-family: Arial, sans-serif;
}
h1 {
text-align: center;
}
.question {
margin-bottom: 20px;
}
.answers {
margin-left: 20px;
}
.answer {
margin-bottom: 10px;
}
.submit-btn {
display: block;
margin: 20px auto;
padding: 10px 20px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
.submit-btn:hover {
background-color: #3E8E41;
}
</style>
</head>
<body>
<h1>在线考试系统</h1>
<div id="questions"></div>
<button class="submit-btn" onclick="submit()">提交</button>
<script>
// 题库
var arr_topic = [
{
question: "1 + 1 = ?",
answers: ["A. 1", "B. 2", "C. 3", "D. 4"],
correctAnswer: "B"
},
{
question: "2 + 2 = ?",
answers: ["A. 2", "B. 3", "C. 4", "D. 5"],
correctAnswer: "C"
},
{
question: "3 + 3 = ?",
answers: ["A. 4", "B. 5", "C. 6", "D. 7"],
correctAnswer: "C"
}
];
// 随机从题库中选取n道题目
var n = 2;
var questions = shuffle(arr_topic).slice(0, n);
// 在页面中显示题目和选项
var questionsDiv = document.getElementById("questions");
for (var i = 0; i < questions.length; i++) {
var question = questions[i];
var questionDiv = document.createElement("div");
questionDiv.className = "question";
questionDiv.innerHTML = "<p>" + question.question + "</p>";
var answersDiv = document.createElement("div");
answersDiv.className = "answers";
for (var j = 0; j < question.answers.length; j++) {
var answer = question.answers[j];
var answerDiv = document.createElement("div");
answerDiv.className = "answer";
answerDiv.innerHTML = "<label><input type='radio' name='answer-" + i + "' value='" + answer.substr(0, 1) + "'> " + answer + "</label>";
answersDiv.appendChild(answerDiv);
}
questionDiv.appendChild(answersDiv);
questionsDiv.appendChild(questionDiv);
}
// 提交答案并计算得分
function submit() {
var score = 0;
for (var i = 0; i < questions.length; i++) {
var question = questions[i];
var selectedAnswer = document.querySelector("input[name='answer-" + i + "']:checked").value;
if (selectedAnswer === question.correctAnswer) {
score++;
}
}
alert("您的得分是:" + score + "分");
}
// 随机打乱数组
function shuffle(arr) {
for (var i = arr.length - 1; i > 0; i--) {
var j = Math.floor(Math.random() * (i + 1));
var temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
return arr;
}
</script>
</body>
</html>
```
该示例中的题库包含了3道数学题,每道题有4个选项,其中只有1个是正确答案。该系统会随机从题库中选取n道题目,然后在页面中显示题目和选项。用户选择答案后,点击“提交”按钮,系统会计算得分并弹出得分提示框。
相关推荐
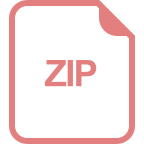
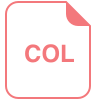
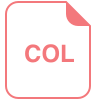
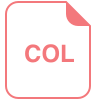
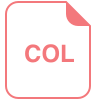
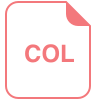









